题目描述 设计一个类模板,实现任意类型数组的排序(升序),至少包括整型、字符、字符串类型。 输入 每组数据首先输入数组的长度 n ,由整型表示,之后顺序输入数组的元素。共输入三组数据,为整型、字符、字符串类型。 输出 输出三组数据升序排序之后的结果
时间: 2024-03-14 17:45:19 浏览: 44
好的,您的问题是如何设计一个类模板来实现任意类型数组的排序(升序),并且至少包括整型、字符、字符串类型。输入三组数据,为整型、字符、字符串类型,并输出它们排序后的结果。
首先,我们需要设计一个类模板,它可以接受任意类型的数组,并能够对其进行排序。我们可以使用模板特化来实现对不同类型的排序。
以下是一个简单的示例:
```cpp
#include <iostream>
#include <algorithm>
#include <string>
using namespace std;
template <typename T>
class SortArray {
public:
SortArray(T* array, int size) : m_array(array), m_size(size) {}
void sort() {
std::sort(m_array, m_array + m_size);
}
void print() {
for (int i = 0; i < m_size; i++) {
cout << m_array[i] << " ";
}
cout << endl;
}
private:
T* m_array;
int m_size;
};
template <>
void SortArray<string>::sort() {
std::sort(m_array, m_array + m_size, [](const string& a, const string& b) {
return a < b;
});
}
int main() {
// 整型数组
int intArray[] = {3, 1, 4, 1, 5, 9, 2, 6, 5, 3};
SortArray<int> intSorter(intArray, sizeof(intArray) / sizeof(int));
intSorter.sort();
intSorter.print();
// 字符数组
char charArray[] = {'h', 'e', 'l', 'l', 'o'};
SortArray<char> charSorter(charArray, sizeof(charArray) / sizeof(char));
charSorter.sort();
charSorter.print();
// 字符串数组
string stringArray[] = {"hello", "world", "this", "is", "a", "test"};
SortArray<string> stringSorter(stringArray, sizeof(stringArray) / sizeof(string));
stringSorter.sort();
stringSorter.print();
return 0;
}
```
在上面的代码中,我们定义了一个 `SortArray` 类模板,它有一个 `sort` 方法和一个 `print` 方法。 `sort` 方法使用 `std::sort` 算法来对数组进行排序,而 `print` 方法用于打印排序后的数组。
我们还定义了一个特化版本,用于对字符串数组进行排序。
在 `main` 函数中,我们使用 `SortArray` 来排序三个不同类型的数组,并打印排序后的结果。
希望这个示例可以帮助您理解如何使用类模板来实现任意类型数组的排序。
相关推荐










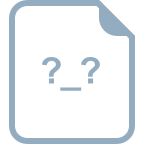
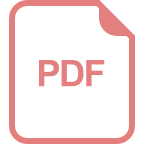
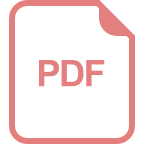