微服务通过feign返回的对象 如何获得
时间: 2023-11-08 09:14:23 浏览: 27
如果你使用 Feign 来进行微服务调用,Feign 会将服务接口封装成一个代理对象,通过这个代理对象来实现远程服务的调用。在 Feign 的默认配置下,Feign 会将服务接口的返回值转换成字符串进行返回,而不是直接返回对象。
如果你想要获得返回的对象,需要进行一些配置。具体来说,你需要在启动类上添加 `@EnableFeignClients` 注解,并且在接口方法上添加 `@RequestMapping` 注解,同时设置 `consumes` 和 `produces` 属性。例如:
```java
@FeignClient(name = "service-provider")
public interface UserFeignClient {
@RequestMapping(value = "/user/{id}", method = RequestMethod.GET,
consumes = "application/json", produces = "application/json")
User getUserById(@PathVariable("id") Long id);
}
```
在这个例子中,`consumes` 和 `produces` 属性都被设置成了 `application/json`,表示接口方法返回的是 JSON 格式的数据。这样,当你调用 `getUserById` 方法时,Feign 就会将返回的 JSON 数据转换成 `User` 对象,然后返回给你。
相关问题
微服务间怎么通过Feign传递信息
在 Feign 中,微服务之间通过 HTTP 请求传递信息。Feign 会将传递的信息即请求参数、请求头等转换成 HTTP 请求,并发送到目标微服务。在请求中可以使用 SpringMVC 的注解(@RequestMapping, @RequestParam等)来定义和传递参数的方式,也可以自定义请求头等。
以下是一个简单的示例:
```java
@FeignClient("service-provider") //指定目标微服务名称
public interface MyFeignClient {
@GetMapping("/user/{id}")
User getUserById(@PathVariable("id") Long id);//使用SpringMVC注解定义参数和请求方式
@PostMapping("/user")
User addUser(@RequestBody User user);//使用SpringMVC注解定义请求方式,并传递请求体
}
```
在上述示例中,通过注解方式定义了两个方法,分别对应了两个 HTTP 请求。Feign 会按照指定的参数和请求方式请求 service-provider 微服务,并将返回结果转换成对应的对象。
feign返回父类对象,缺失子类对象
Feign 是一个基于 Java 的 HTTP 客户端,它允许您使用注解的方式来定义 HTTP 请求和响应的接口,在 Spring Cloud 微服务架构中广泛应用。关于您提到的问题,可能是由于 Feign 在反序列化返回对象时,无法正确地识别该对象的子类类型。
为了解决这个问题,您需要在 Feign 的请求接口中使用 `@RequestLine` 注解来指定请求的 HTTP 方法和路径,然后在返回类型中使用泛型来指定实际返回的类型。例如:
```java
public interface MyFeignClient {
@RequestLine("GET /api/your/path")
MyResponse<MyEntity> getMyEntity();
}
```
其中,`MyResponse` 是一个封装了 HTTP 响应结果的通用类,而 `MyEntity` 则是您具体的实体类,如果您的实体类有多个子类,那么您需要在 `MyResponse` 中添加一个字段来表示实际的子类类型。例如:
```java
public class MyResponse<T> {
private String type;
private T data;
// getter and setter methods
}
```
在这种情况下,您需要在服务端返回的 JSON 数据中包含一个 `type` 字段,用来表示实际的子类类型。然后在 Feign 客户端的 `MyResponse` 中解析该字段,并根据实际的子类类型来反序列化 `data` 字段。如果服务端返回的 JSON 数据中没有包含 `type` 字段,那么 Feign 将无法正确地识别对象的子类类型,从而导致缺失子类对象的问题。
相关推荐
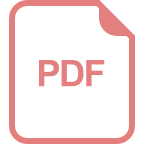
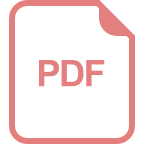












