你可以帮我写一个关于STM32F407VET6 的TFTLCD初始化程序吗
时间: 2023-08-28 10:03:20 浏览: 114
当然可以,以下是一个简单的TFTLCD初始化程序:
```c
#include "stm32f4xx.h"
#include "tftlcd.h"
// 定义LCD控制引脚
#define LCD_CS_PIN GPIO_Pin_2 // PD2
#define LCD_DC_PIN GPIO_Pin_3 // PD3
#define LCD_RST_PIN GPIO_Pin_4 // PD4
// 定义LCD控制引脚所在的GPIO端口
#define LCD_GPIO_PORT GPIOD
// 定义LCD控制引脚所在的时钟
#define LCD_GPIO_CLK RCC_AHB1Periph_GPIOD
// 定义LCD初始化函数
void TFTLCD_Init(void)
{
// 初始化GPIO时钟
RCC_AHB1PeriphClockCmd(LCD_GPIO_CLK, ENABLE);
// 设置控制引脚为输出模式
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin = LCD_CS_PIN | LCD_DC_PIN | LCD_RST_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_OUT;
GPIO_InitStructure.GPIO_OType = GPIO_OType_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_100MHz;
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_NOPULL;
GPIO_Init(LCD_GPIO_PORT, &GPIO_InitStructure);
// 复位LCD
GPIO_ResetBits(LCD_GPIO_PORT, LCD_RST_PIN);
Delay(20);
GPIO_SetBits(LCD_GPIO_PORT, LCD_RST_PIN);
Delay(20);
// 发送命令进行初始化
TFTLCD_WriteCommand(0xEF);
TFTLCD_WriteData(0x03);
TFTLCD_WriteData(0x80);
TFTLCD_WriteData(0x02);
TFTLCD_WriteCommand(0xCF);
TFTLCD_WriteData(0x00);
TFTLCD_WriteData(0xC1);
TFTLCD_WriteData(0x30);
TFTLCD_WriteCommand(0xED);
TFTLCD_WriteData(0x64);
TFTLCD_WriteData(0x03);
TFTLCD_WriteData(0x12);
TFTLCD_WriteData(0x81);
TFTLCD_WriteCommand(0xE8);
TFTLCD_WriteData(0x85);
TFTLCD_WriteData(0x00);
TFTLCD_WriteData(0x78);
TFTLCD_WriteCommand(0xCB);
TFTLCD_WriteData(0x39);
TFTLCD_WriteData(0x2C);
TFTLCD_WriteData(0x00);
TFTLCD_WriteData(0x34);
TFTLCD_WriteData(0x02);
TFTLCD_WriteCommand(0xF7);
TFTLCD_WriteData(0x20);
TFTLCD_WriteCommand(0xEA);
TFTLCD_WriteData(0x00);
TFTLCD_WriteData(0x00);
TFTLCD_WriteCommand(0xC0); // Power control
TFTLCD_WriteData(0x1B); // VRH[5:0]
TFTLCD_WriteCommand(0xC1); // Power control
TFTLCD_WriteData(0x01); // SAP[2:0];BT[3:0]
TFTLCD_WriteCommand(0xC5); // VCM control
TFTLCD_WriteData(0x30); // VCM[5:0]
TFTLCD_WriteData(0x30); // VCM[5:0]
TFTLCD_WriteCommand(0xC7); // VCM control2
TFTLCD_WriteData(0XB7);
TFTLCD_WriteCommand(0x36); // Memory Access Control
TFTLCD_WriteData(0x08);
TFTLCD_WriteCommand(0x3A);
TFTLCD_WriteData(0x55);
TFTLCD_WriteCommand(0xB1);
TFTLCD_WriteData(0x00);
TFTLCD_WriteData(0x1A);
TFTLCD_WriteCommand(0xB6); // Display Function Control
TFTLCD_WriteData(0x0A);
TFTLCD_WriteData(0xA2);
TFTLCD_WriteCommand(0xF2); // 3Gamma Function Disable
TFTLCD_WriteData(0x00);
TFTLCD_WriteCommand(0x26); // Gamma curve selected
TFTLCD_WriteData(0x01);
TFTLCD_WriteCommand(0xE0); // Set Gamma
TFTLCD_WriteData(0x0F);
TFTLCD_WriteData(0x2A);
TFTLCD_WriteData(0x28);
TFTLCD_WriteData(0x08);
TFTLCD_WriteData(0x0E);
TFTLCD_WriteData(0x08);
TFTLCD_WriteData(0x54);
TFTLCD_WriteData(0XA9);
TFTLCD_WriteData(0x43);
TFTLCD_WriteData(0x0A);
TFTLCD_WriteData(0x0F);
TFTLCD_WriteData(0x00);
TFTLCD_WriteData(0x00);
TFTLCD_WriteData(0x00);
TFTLCD_WriteData(0x00);
TFTLCD_WriteCommand(0XE1); // Set Gamma
TFTLCD_WriteData(0x00);
TFTLCD_WriteData(0x15);
TFTLCD_WriteData(0x17);
TFTLCD_WriteData(0x07);
TFTLCD_WriteData(0x11);
TFTLCD_WriteData(0x06);
TFTLCD_WriteData(0x2B);
TFTLCD_WriteData(0x56);
TFTLCD_WriteData(0x3C);
TFTLCD_WriteData(0x05);
TFTLCD_WriteData(0x10);
TFTLCD_WriteData(0x0F);
TFTLCD_WriteData(0x3F);
TFTLCD_WriteData(0x3F);
TFTLCD_WriteData(0x0F);
TFTLCD_WriteCommand(0x11); // Exit Sleep
Delay(120);
TFTLCD_WriteCommand(0x29); // Display on
}
// 定义LCD写命令函数
void TFTLCD_WriteCommand(uint16_t cmd)
{
GPIO_ResetBits(LCD_GPIO_PORT, LCD_DC_PIN);
GPIO_ResetBits(LCD_GPIO_PORT, LCD_CS_PIN);
SPI_I2S_SendData(SPI5, cmd);
while (SPI_I2S_GetFlagStatus(SPI5, SPI_I2S_FLAG_BSY) != RESET);
GPIO_SetBits(LCD_GPIO_PORT, LCD_CS_PIN);
}
// 定义LCD写数据函数
void TFTLCD_WriteData(uint16_t data)
{
GPIO_SetBits(LCD_GPIO_PORT, LCD_DC_PIN);
GPIO_ResetBits(LCD_GPIO_PORT, LCD_CS_PIN);
SPI_I2S_SendData(SPI5, data);
while (SPI_I2S_GetFlagStatus(SPI5, SPI_I2S_FLAG_BSY) != RESET);
GPIO_SetBits(LCD_GPIO_PORT, LCD_CS_PIN);
}
// 定义延时函数
void Delay(__IO uint32_t nCount)
{
while(nCount--);
}
```
注:此代码仅供参考,具体实现需要根据硬件和屏幕规格进行修改。
阅读全文
相关推荐
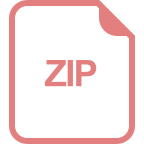
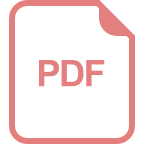
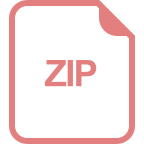
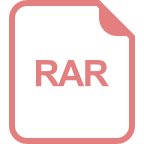
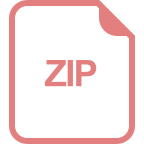
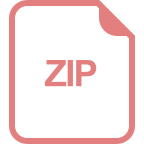
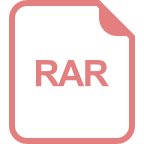
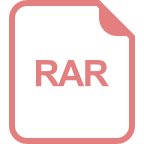
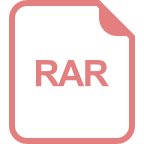
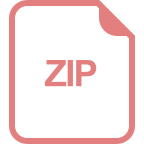
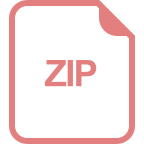
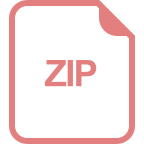
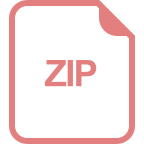
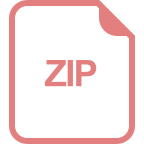
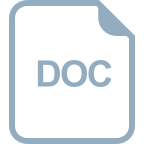
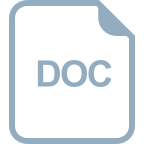
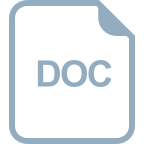