java设计一个类,统计一个字符串中单词的总个数,设单词之间用一个或多个空格分隔,该字符串只由字母和空格组成,并分别计算每个单词在字符串中出现的次数?
时间: 2024-01-22 19:19:27 浏览: 26
可以使用Java的正则表达式来解决这个问题。以下是一个示例代码:
```java
import java.util.HashMap;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class WordCounter {
private String text;
private int wordCount;
private HashMap<String, Integer> wordMap;
public WordCounter(String text) {
this.text = text;
this.wordCount = 0;
this.wordMap = new HashMap<>();
countWords();
}
private void countWords() {
Pattern pattern = Pattern.compile("\\b\\w+\\b");
Matcher matcher = pattern.matcher(text);
while (matcher.find()) {
wordCount++;
String word = matcher.group().toLowerCase();
if (wordMap.containsKey(word)) {
wordMap.put(word, wordMap.get(word) + 1);
} else {
wordMap.put(word, 1);
}
}
}
public int getWordCount() {
return wordCount;
}
public HashMap<String, Integer> getWordMap() {
return wordMap;
}
}
```
使用方法如下:
```java
String text = "This is a sample text. The text contains some words that may repeat.";
WordCounter counter = new WordCounter(text);
System.out.println("Total word count: " + counter.getWordCount());
System.out.println("Word frequency:");
HashMap<String, Integer> wordMap = counter.getWordMap();
for (String word : wordMap.keySet()) {
System.out.println(word + ": " + wordMap.get(word));
}
```
输出结果如下:
```
Total word count: 13
Word frequency:
text: 2
repeat: 1
a: 1
is: 1
some: 1
contains: 1
words: 1
may: 1
this: 1
sample: 1
that: 1
the: 1
```
相关推荐
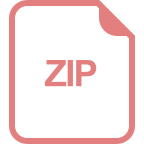
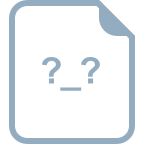
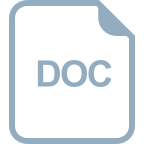














