帮我写一段I2C一主多从机 读写控制 C语言程序
时间: 2023-10-05 12:14:42 浏览: 34
好的,下面是一个简单的示例程序,演示了如何使用I2C进行一主多从机的读写控制:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdint.h>
#include <unistd.h>
#include <fcntl.h>
#include <sys/ioctl.h>
#include <linux/i2c-dev.h>
#define I2C_BUS "/dev/i2c-1"
#define I2C_SLAVE_ADDR1 0x50
#define I2C_SLAVE_ADDR2 0x51
int main()
{
int fd;
uint8_t data1[] = {0x01, 0x02, 0x03, 0x04};
uint8_t data2[] = {0x05, 0x06, 0x07, 0x08};
uint8_t read_data1[4];
uint8_t read_data2[4];
// 打开I2C总线
fd = open(I2C_BUS, O_RDWR);
if (fd < 0) {
perror("Failed to open I2C bus");
exit(1);
}
// 设置从机地址1
if (ioctl(fd, I2C_SLAVE, I2C_SLAVE_ADDR1) < 0) {
perror("Failed to set I2C slave address1");
exit(1);
}
// 写入数据到从机1
if (write(fd, data1, sizeof(data1)) != sizeof(data1)) {
perror("Failed to write data to I2C slave1");
exit(1);
}
// 读取从机1返回的数据
if (read(fd, read_data1, sizeof(read_data1)) != sizeof(read_data1)) {
perror("Failed to read data from I2C slave1");
exit(1);
}
// 设置从机地址2
if (ioctl(fd, I2C_SLAVE, I2C_SLAVE_ADDR2) < 0) {
perror("Failed to set I2C slave address2");
exit(1);
}
// 写入数据到从机2
if (write(fd, data2, sizeof(data2)) != sizeof(data2)) {
perror("Failed to write data to I2C slave2");
exit(1);
}
// 读取从机2返回的数据
if (read(fd, read_data2, sizeof(read_data2)) != sizeof(read_data2)) {
perror("Failed to read data from I2C slave2");
exit(1);
}
// 关闭I2C总线
close(fd);
// 打印读取到的数据
printf("Read data from I2C slave1: ");
for (int i = 0; i < sizeof(read_data1); i++) {
printf("%02x ", read_data1[i]);
}
printf("\n");
printf("Read data from I2C slave2: ");
for (int i = 0; i < sizeof(read_data2); i++) {
printf("%02x ", read_data2[i]);
}
printf("\n");
return 0;
}
```
在上面的示例程序中,我们打开I2C总线并设置从机地址1,然后写入数据到从机1,并读取从机1返回的数据。接着,我们设置从机地址2,写入数据到从机2,并读取从机2返回的数据。最后,我们关闭I2C总线并打印读取到的数据。
相关推荐
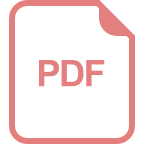
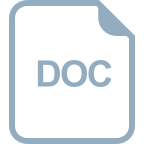
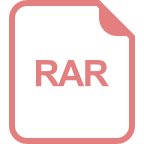














