使用vue,将商品详情页的数据存储到pinia,购物车页面读取pinia里的数据并显示
时间: 2023-10-25 13:11:47 浏览: 40
首先,需要安装 Pinia:
```
npm install pinia
```
然后,在 Vue 3 的入口文件中引入和创建 Pinia 实例:
```javascript
import { createApp } from 'vue'
import { createPinia } from 'pinia'
import App from './App.vue'
const app = createApp(App)
// 创建 Pinia 实例
const pinia = createPinia()
// 将 Pinia 实例挂载到应用上
app.use(pinia)
// 启动应用
app.mount('#app')
```
接下来,我们在 Pinia 中定义 `ProductStore` 和 `CartStore`:
```javascript
import { defineStore } from 'pinia'
export const ProductStore = defineStore('product', {
state: () => ({
products: []
}),
actions: {
fetchProducts() {
// 异步获取商品数据
// ...
// 获取到商品数据后,将数据存储到 state 中
this.products = [ /* 商品数据 */ ]
}
}
})
export const CartStore = defineStore('cart', {
state: () => ({
cartItems: []
}),
actions: {
addItemToCart(item) {
// 添加商品到购物车
// ...
// 添加成功后,将购物车数据更新到 state 中
this.cartItems.push(item)
}
}
})
```
在商品详情页中,我们需要获取商品数据并将数据存储到 `ProductStore` 中:
```html
<template>
<div>
<h1>{{ product.name }}</h1>
<p>{{ product.description }}</p>
<button @click="addToCart">加入购物车</button>
</div>
</template>
<script>
import { defineComponent, computed } from 'vue'
import { useRoute } from 'vue-router'
import { useStore } from 'pinia'
import { ProductStore } from '@/stores'
export default defineComponent({
setup() {
const route = useRoute()
const store = useStore(ProductStore)
const productId = route.params.id
// 获取商品数据
store.fetchProducts()
// 根据商品 ID 获取商品详情
const product = computed(() => {
return store.products.find(item => item.id === productId)
})
// 添加商品到购物车
const addToCart = () => {
const item = {
id: product.value.id,
name: product.value.name,
price: product.value.price,
quantity: 1
}
store.cart.addItemToCart(item)
}
return {
product,
addToCart
}
}
})
</script>
```
在购物车页面中,我们需要读取 `CartStore` 中的数据并显示:
```html
<template>
<div>
<h1>购物车</h1>
<ul>
<li v-for="item in cartItems" :key="item.id">
<p>{{ item.name }}</p>
<p>{{ item.price }}</p>
<p>{{ item.quantity }}</p>
</li>
</ul>
</div>
</template>
<script>
import { defineComponent } from 'vue'
import { useStore } from 'pinia'
import { CartStore } from '@/stores'
export default defineComponent({
setup() {
const store = useStore(CartStore)
// 获取购物车数据
const cartItems = store.cartItems
return {
cartItems
}
}
})
</script>
```
最后,我们需要在路由中定义商品详情页和购物车页面的路由:
```javascript
import { createRouter, createWebHistory } from 'vue-router'
import ProductDetail from '@/views/ProductDetail.vue'
import ShoppingCart from '@/views/ShoppingCart.vue'
const routes = [
{
path: '/product/:id',
name: 'ProductDetail',
component: ProductDetail
},
{
path: '/shopping-cart',
name: 'ShoppingCart',
component: ShoppingCart
}
]
const router = createRouter({
history: createWebHistory(),
routes
})
export default router
```
这样,我们就完成了将商品详情页的数据存储到 Pinia,购物车页面读取 Pinia 里的数据并显示的流程。
相关推荐
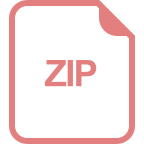














