用c++设计一个折线类
时间: 2023-05-30 15:05:46 浏览: 66
折线类(Line)可以包含以下属性和方法:
属性:
- 点数组(points):包含所有构成折线的点坐标。
方法:
- 构造函数(Line):用点数组初始化折线对象。
- 添加点(addPoint):在折线末尾添加一个点。
- 删除点(removePoint):删除指定位置的点。
- 获取点(getPoint):获取指定位置的点。
- 获取点数(getPointCount):获取折线中点的数量。
- 获取长度(getLength):计算折线的总长度。
- 获取起点(getStartPoint):获取折线的起点。
- 获取终点(getEndPoint):获取折线的终点。
- 移动折线(move):将折线平移一定的距离。
以下是折线类的示例代码:
```c
#include <stdio.h>
#include <math.h>
// 定义点结构体
typedef struct Point {
double x;
double y;
} Point;
// 折线类
typedef struct Line {
Point* points; // 点数组
int count; // 点数量
} Line;
// 构造函数:用点数组初始化折线对象
void Line_init(Line* this, Point* points, int count) {
this->points = points;
this->count = count;
}
// 在折线末尾添加一个点
void Line_addPoint(Line* this, Point point) {
this->points[this->count++] = point;
}
// 删除指定位置的点
void Line_removePoint(Line* this, int index) {
if (index >= 0 && index < this->count) {
for (int i = index; i < this->count - 1; i++) {
this->points[i] = this->points[i + 1];
}
this->count--;
}
}
// 获取指定位置的点
Point Line_getPoint(Line* this, int index) {
Point point = {0, 0};
if (index >= 0 && index < this->count) {
point = this->points[index];
}
return point;
}
// 获取折线中点的数量
int Line_getPointCount(Line* this) {
return this->count;
}
// 计算两点之间的距离
double distance(Point a, Point b) {
return sqrt(pow(a.x - b.x, 2) + pow(a.y - b.y, 2));
}
// 计算折线的总长度
double Line_getLength(Line* this) {
double length = 0;
for (int i = 0; i < this->count - 1; i++) {
length += distance(this->points[i], this->points[i + 1]);
}
return length;
}
// 获取折线的起点
Point Line_getStartPoint(Line* this) {
return this->points[0];
}
// 获取折线的终点
Point Line_getEndPoint(Line* this) {
return this->points[this->count - 1];
}
// 将折线平移一定的距离
void Line_move(Line* this, double dx, double dy) {
for (int i = 0; i < this->count; i++) {
this->points[i].x += dx;
this->points[i].y += dy;
}
}
int main() {
Point points[] = {{0, 0}, {1, 1}, {2, 3}, {4, 4}};
Line line;
Line_init(&line, points, 4);
printf("Length: %f\n", Line_getLength(&line));
printf("Start point: (%f, %f)\n", Line_getStartPoint(&line).x, Line_getStartPoint(&line).y);
printf("End point: (%f, %f)\n", Line_getEndPoint(&line).x, Line_getEndPoint(&line).y);
Line_move(&line, 1, 2);
printf("New start point: (%f, %f)\n", Line_getStartPoint(&line).x, Line_getStartPoint(&line).y);
return 0;
}
```
相关推荐
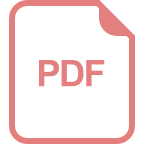
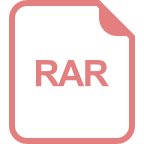














