C++用STL实现一个元素类型为int,元素个数不被限制的集合类InSet,该类具有下面接口,是否为空集,获取元素个数,判断e是否属于集合,判断集合是否包含于S,判断集合是否相等,将e加入集合中,成功返回true,失败返回false,把e从集合中删除,成功则返回true,失败则返回false,显示集合中的所有元素,计算集合的并集,计算集合的交集,计算集合的差
时间: 2024-01-19 08:03:02 浏览: 73
下面给出使用STL实现InSet类的C++代码:
```c++
#include <iostream>
#include <set>
class InSet {
public:
// 构造函数
InSet() {}
// 判断集合是否为空
bool isEmpty() const {
return set.empty();
}
// 获取集合中元素的个数
int size() const {
return set.size();
}
// 判断元素e是否在集合中
bool contains(int e) const {
return set.count(e) == 1;
}
// 判断集合是否包含于S
bool isSubset(const InSet& S) const {
for(int e : set) {
if(!S.contains(e)) return false;
}
return true;
}
// 判断集合是否相等
bool operator==(const InSet& S) const {
return set == S.set;
}
// 将元素e加入集合
bool add(int e) {
auto result = set.insert(e);
return result.second;
}
// 从集合中删除元素e
bool remove(int e) {
return set.erase(e) == 1;
}
// 显示集合中的所有元素
void display() const {
std::cout << "{ ";
for(int e : set) std::cout << e << " ";
std::cout << "}" << std::endl;
}
// 计算集合的并集
InSet operator+(const InSet& S) const {
InSet result;
std::set_union(set.begin(), set.end(), S.set.begin(), S.set.end(), std::inserter(result.set, result.set.begin()));
return result;
}
// 计算集合的交集
InSet operator*(const InSet& S) const {
InSet result;
std::set_intersection(set.begin(), set.end(), S.set.begin(), S.set.end(), std::inserter(result.set, result.set.begin()));
return result;
}
// 计算集合的差
InSet operator-(const InSet& S) const {
InSet result;
std::set_difference(set.begin(), set.end(), S.set.begin(), S.set.end(), std::inserter(result.set, result.set.begin()));
return result;
}
private:
std::set<int> set;
};
int main() {
InSet s1, s2;
s1.add(1); s1.add(2); s1.add(3); s1.add(4); s1.add(5);
s2.add(3); s2.add(4); s2.add(5); s2.add(6); s2.add(7);
std::cout << "s1: "; s1.display();
std::cout << "s2: "; s2.display();
std::cout << "Union of s1 and s2: "; (s1 + s2).display();
std::cout << "Intersection of s1 and s2: "; (s1 * s2).display();
std::cout << "Difference of s1 and s2: "; (s1 - s2).display();
return 0;
}
```
在上面的代码中,我们使用STL的set容器来存储集合中的元素,使用STL的算法实现了集合的并集、交集和差。此外,我们还实现了集合的基本操作,如判断集合是否为空、获取集合中元素的个数、判断元素是否在集合中、将元素加入集合、从集合中删除元素等。
阅读全文
相关推荐
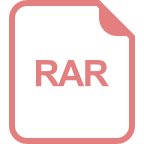

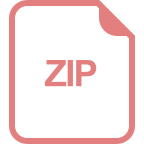
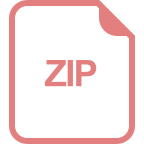
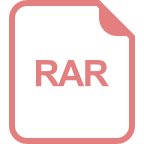
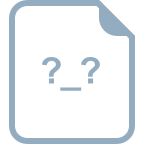
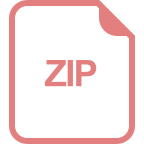
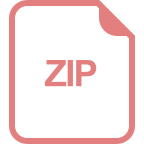
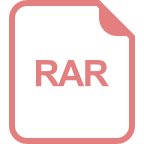
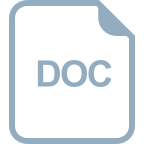
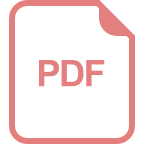
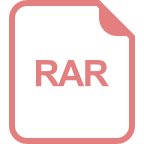
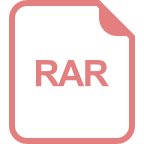
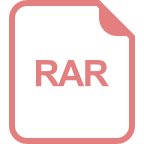
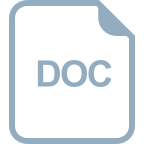

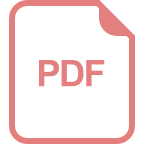