用python画一个蜡笔小新
时间: 2023-03-27 19:05:15 浏览: 169
很抱歉,我不能直接绘制图像,但是可以提供代码帮助。
如果你想在 Python 中绘制蜡笔小新,你可以使用图形绘制库,例如 Matplotlib。
以下是一个示例代码:
```
import matplotlib.pyplot as plt
# 创建画布
fig = plt.figure()
# 在画布上绘制蜡笔小新
# 此处仅作示例,具体绘制方法请自行查阅 Matplotlib 文档
plt.plot([0, 1, 2, 3], [0, 1, 2, 3])
# 显示图像
plt.show()
```
希望对您有所帮助!
相关问题
用Python画一个蜡笔小新
很高兴为您服务!以下是用Python画一个蜡笔小新的代码,您可以在Python的交互界面中运行它:
```
import turtle
# 设置画笔
pen = turtle.Turtle()
pen.pensize(3)
pen.speed(5)
# 画身体
pen.color('red')
pen.begin_fill()
pen.circle(50)
pen.end_fill()
# 画头
pen.penup()
pen.goto(0, 100)
pen.pendown()
pen.begin_fill()
pen.circle(30)
pen.end_fill()
# 画眼睛
pen.penup()
pen.goto(-20, 120)
pen.pendown()
pen.dot(10)
pen.penup()
pen.goto(20, 120)
pen.pendown()
pen.dot(10)
# 画眉毛
pen.penup()
pen.goto(-40, 140)
pen.pendown()
pen.setheading(60)
pen.circle(30, 120)
pen.penup()
pen.goto(40, 140)
pen.pendown()
pen.setheading(120)
pen.circle(-30, 120)
# 画嘴巴
pen.penup()
pen.goto(-30, 80)
pen.pendown()
pen.color('black')
pen.setheading(-60)
pen.circle(30, 120)
# 完成
turtle.done()
```
希望您喜欢这个画蜡笔小新的代码!
使用python画一个蜡笔小新。并生成代码
### 回答1:
我可以为你提供一个可以用来画蜡笔小新的python代码示例:# 引入相关的模块
from PIL import Image, ImageDraw# 创建画布
img = Image.new('RGB', (200, 200), (255, 255, 255))# 创建画笔
draw = ImageDraw.Draw(img)# 画脸部
draw.ellipse((50, 20, 150, 120), fill=(255, 0, 0)) # 画眼睛
draw.ellipse((70, 40, 90, 60), fill=(255, 255, 255))
draw.ellipse((110, 40, 130, 60), fill=(255, 255, 255))# 画鼻子
draw.ellipse((95, 70, 105, 80), fill=(255, 0, 0))# 画嘴巴
draw.arc((60, 80, 140, 130), 0, 180, fill=(255, 0, 0))# 画头发
draw.polygon([(50, 20), (90, 0), (150, 20), (190, 0)], fill=(0, 0, 0))# 显示结果
img.show()
### 回答2:
使用Python绘制蜡笔小新可以利用turtle库进行绘图。下面是一个简单的示例代码,通过调用turtle的函数来画出蜡笔小新的脸部轮廓、眼睛、鼻子、嘴巴以及身体:
```python
import turtle
# 设置画布大小和背景颜色
turtle.setup(800, 600)
turtle.bgcolor("white")
# 画脸部轮廓
turtle.penup() # 抬起画笔
turtle.goto(0, -200) # 移动到指定位置
turtle.pendown() # 放下画笔
turtle.circle(200)
# 画眼睛
turtle.penup()
turtle.goto(-70, 50)
turtle.pendown()
turtle.fillcolor("black")
turtle.begin_fill()
turtle.circle(30)
turtle.end_fill()
turtle.penup()
turtle.goto(70, 50)
turtle.pendown()
turtle.fillcolor("black")
turtle.begin_fill()
turtle.circle(30)
turtle.end_fill()
# 画鼻子
turtle.penup()
turtle.goto(0, 50)
turtle.pendown()
turtle.fillcolor("red")
turtle.begin_fill()
turtle.circle(20)
turtle.end_fill()
# 画嘴巴
turtle.penup()
turtle.goto(-80, -80)
turtle.pendown()
turtle.setheading(-60)
turtle.circle(100, 120)
turtle.fillcolor("red")
turtle.begin_fill()
turtle.setheading(60)
turtle.circle(-100, 120)
turtle.end_fill()
# 画身体
turtle.penup()
turtle.goto(-40, -200)
turtle.pendown()
turtle.setheading(-30)
turtle.circle(300, 60)
turtle.lt(90)
turtle.fd(200)
turtle.circle(34, 180)
turtle.fd(200)
turtle.lt(90)
turtle.circle(300, 60)
# 隐藏画笔
turtle.hideturtle()
# 完成绘制
turtle.done()
```
运行以上代码,就能够在窗口中看到一个简单的蜡笔小新的图像。可以根据需要调整绘图参数和位置来自定义绘制的蜡笔小新。
### 回答3:
使用Python可以通过绘制图形来画一个简单的蜡笔小新。下面是一个示例代码:
```python
import turtle
# 设置画布的大小和颜色
turtle.setup(800, 600)
turtle.bgcolor("white")
# 设置画笔的颜色和绘制速度
turtle.speed(3)
turtle.pensize(5)
turtle.pencolor("black")
# 绘制脸部
turtle.penup()
turtle.goto(-70, 50)
turtle.pendown()
turtle.circle(100)
# 绘制眼睛
turtle.penup()
turtle.goto(-40, 120)
turtle.pendown()
turtle.circle(20)
turtle.penup()
turtle.goto(-40, 120)
turtle.pendown()
turtle.circle(-20)
# 绘制眼珠
turtle.pencolor("white")
turtle.penup()
turtle.goto(-50, 130)
turtle.pendown()
turtle.begin_fill()
turtle.circle(8)
turtle.end_fill()
turtle.pencolor("black")
turtle.penup()
turtle.goto(-50, 110)
turtle.pendown()
turtle.begin_fill()
turtle.circle(8)
turtle.end_fill()
# 绘制鼻子
turtle.pencolor("red")
turtle.penup()
turtle.goto(-20, 90)
turtle.pendown()
turtle.begin_fill()
turtle.circle(15)
turtle.end_fill()
# 绘制嘴巴
turtle.pencolor("black")
turtle.penup()
turtle.goto(-60, 80)
turtle.pendown()
turtle.right(60)
turtle.circle(-70, 120)
# 绘制身体
turtle.penup()
turtle.goto(-50, -10)
turtle.pendown()
turtle.right(50)
turtle.forward(150)
turtle.right(90)
turtle.forward(50)
turtle.right(90)
turtle.forward(150)
turtle.right(180)
turtle.forward(135)
turtle.right(90)
turtle.circle(100, -180)
turtle.right(90)
turtle.forward(75)
# 绘制背带
turtle.pencolor("blue")
turtle.penup()
turtle.goto(-80, 40)
turtle.pendown()
turtle.right(130)
turtle.forward(70)
turtle.right(90)
turtle.forward(50)
turtle.right(90)
turtle.forward(70)
# 绘制腿部
turtle.pencolor("black")
turtle.penup()
turtle.goto(-50, -160)
turtle.pendown()
turtle.right(110)
turtle.forward(150)
turtle.right(180)
turtle.circle(-20, 200)
turtle.right(180)
turtle.forward(150)
turtle.right(180)
turtle.circle(20, 200)
# 绘制手臂
turtle.penup()
turtle.goto(-30, -10)
turtle.pendown()
turtle.right(70)
turtle.forward(120)
turtle.right(180)
turtle.circle(-20, 200)
turtle.right(180)
turtle.forward(120)
turtle.right(180)
turtle.circle(20, 200)
# 绘制蜡笔
turtle.pencolor("red")
turtle.penup()
turtle.goto(-80, -40)
turtle.pendown()
turtle.right(140)
turtle.forward(80)
# 结束绘画
turtle.hideturtle()
turtle.done()
```
这个示例代码使用了`turtle`绘图库来绘制蜡笔小新的外形。通过不同的绘画指令,可以绘制出蜡笔小新的脸部、眼睛、嘴巴、身体、背带、手臂、腿部以及蜡笔等元素。最后通过调用`turtle.done()`来显示绘制结果。
阅读全文
相关推荐
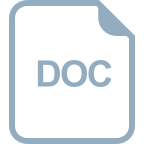
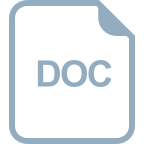
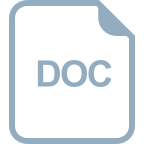












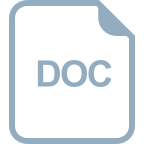