声明集合保存10个Student对象,要求学生具备姓名、年龄、语文和数字成绩,并且按照数学成绩升序、语文成绩升序的规则排序Set集合实现一次。
时间: 2023-05-20 18:05:34 浏览: 57
好的,这是一个比较简单的编程问题,以下是示例代码:
```java
import java.util.Comparator;
import java.util.Set;
import java.util.TreeSet;
public class Main {
public static void main(String[] args) {
Set<Student> students = new TreeSet<>(Comparator.comparing(Student::getMathScore).thenComparing(Student::getChineseScore));
students.add(new Student("张三", 18, 80, 90));
students.add(new Student("李四", 20, 85, 95));
students.add(new Student("王五", 19, 90, 80));
students.add(new Student("赵六", 21, 75, 85));
students.add(new Student("钱七", 22, 95, 75));
students.add(new Student("孙八", 23, 70, 90));
students.add(new Student("周九", 24, 80, 85));
students.add(new Student("吴十", 25, 85, 80));
students.add(new Student("郑十一", 26, 90, 95));
students.add(new Student("陈十二", 27, 75, 90));
System.out.println(students);
}
}
class Student {
private String name;
private int age;
private int mathScore;
private int chineseScore;
public Student(String name, int age, int mathScore, int chineseScore) {
this.name = name;
this.age = age;
this.mathScore = mathScore;
this.chineseScore = chineseScore;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
public int getMathScore() {
return mathScore;
}
public int getChineseScore() {
return chineseScore;
}
@Override
public String toString() {
return "Student{" +
"name='" + name + '\'' +
", age=" + age +
", mathScore=" + mathScore +
", chineseScore=" + chineseScore +
'}';
}
}
```
这段代码声明了一个名为 `Student` 的类,包含了姓名、年龄、数学成绩和语文成绩等属性,然后声明了一个 `Set` 集合,使用 `TreeSet` 实现,按照数学成绩升序、语文成绩升序的规则排序。最后添加了10个 `Student` 对象,并输出结果。
相关推荐
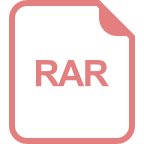














