利用pygame模块实现飞机大战附加功能如下: (1) 实现碰撞检测(可以消灭敌机,也可以被敌机消灭) (2) 随机出现云朵在天空中漂浮 (3) 随机出现甜甜圈进行能量补给(生命力或子弹类型发生变化) (4) 有GAME OVER条件控制处理
时间: 2023-10-24 08:08:39 浏览: 57
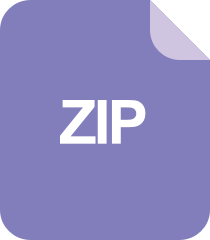
利用python内置的pygame模块实现飞机大战游戏
好的,以下是利用pygame模块实现飞机大战附加功能的代码:
```python
import pygame
import random
# 初始化pygame
pygame.init()
# 游戏界面大小
screen = pygame.display.set_mode((480, 700))
# 游戏标题
pygame.display.set_caption("飞机大战")
# 加载背景图片
bg = pygame.image.load("images/background.png")
# 加载飞机图片
player_img = pygame.image.load("images/me1.png")
# 加载敌机图片
enemy_img = pygame.image.load("images/enemy1.png")
# 加载云朵图片
cloud_img = pygame.image.load("images/cloud.png")
# 加载甜甜圈图片
supply_img = pygame.image.load("images/supply.png")
# 加载音效
bullet_sound = pygame.mixer.Sound("sounds/bullet.wav")
supply_sound = pygame.mixer.Sound("sounds/supply.wav")
enemy_down_sound = pygame.mixer.Sound("sounds/enemy_down.wav")
game_over_sound = pygame.mixer.Sound("sounds/game_over.wav")
# 设置字体
font = pygame.font.SysFont("simhei", 25)
# 飞机尺寸
player_width = player_img.get_width()
player_height = player_img.get_height()
# 敌机尺寸
enemy_width = enemy_img.get_width()
enemy_height = enemy_img.get_height()
# 云朵尺寸
cloud_width = cloud_img.get_width()
cloud_height = cloud_img.get_height()
# 甜甜圈尺寸
supply_width = supply_img.get_width()
supply_height = supply_img.get_height()
# 定义子弹列表
bullet_list = []
# 定义敌机列表
enemy_list = []
# 定义云朵列表
cloud_list = []
# 定义甜甜圈列表
supply_list = []
# 定义能量类型:0表示普通子弹,1表示双倍子弹
bullet_type = 0
# 定义生命值
life = 3
# 定义得分
score = 0
# 定义游戏结束标志
game_over = False
# 定义时间变量
clock = pygame.time.Clock()
# 定义飞机类
class Player(pygame.sprite.Sprite):
def __init__(self):
super().__init__()
self.image = player_img
self.rect = self.image.get_rect()
self.rect.left = (480 - player_width) // 2
self.rect.top = 500
# 移动飞机
def move(self, x, y):
self.rect.left += x
self.rect.top += y
# 发射子弹
def shoot(self):
bullet_sound.play()
bullet = Bullet(self.rect.left + player_width // 2 - 3, self.rect.top - 20)
bullet_list.append(bullet)
# 碰撞检测
def collide(self, enemies):
for enemy in enemies:
if pygame.sprite.collide_rect(self, enemy):
enemy.down()
self.down()
break
# 被击落
def down(self):
global life
life -= 1
if life == 0:
game_over_sound.play()
global game_over
game_over = True
else:
self.rect.left = (480 - player_width) // 2
self.rect.top = 500
# 定义子弹类
class Bullet(pygame.sprite.Sprite):
def __init__(self, x, y):
super().__init__()
if bullet_type == 0:
self.image = pygame.image.load("images/bullet1.png")
else:
self.image = pygame.image.load("images/bullet2.png")
self.rect = self.image.get_rect()
self.rect.left = x
self.rect.top = y
# 移动子弹
def move(self):
self.rect.top -= 10
# 是否越界
def out_of_range(self):
if self.rect.top < -self.rect.height:
return True
else:
return False
# 碰撞检测
def collide(self, enemies):
for enemy in enemies:
if pygame.sprite.collide_rect(self, enemy):
enemy.down()
bullet_list.remove(self)
break
# 定义敌机类
class Enemy(pygame.sprite.Sprite):
def __init__(self):
super().__init__()
self.image = enemy_img
self.rect = self.image.get_rect()
self.rect.left = random.randint(0, 480 - enemy_width)
self.rect.top = -enemy_height
# 移动敌机
def
阅读全文
相关推荐
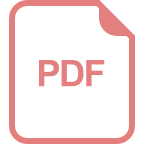
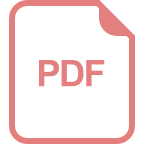
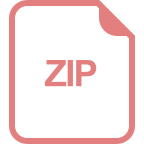
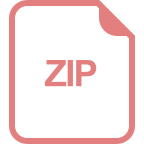
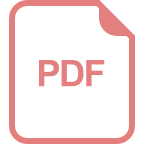
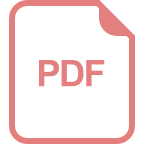
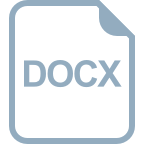
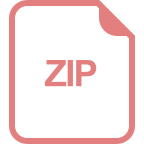
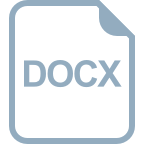
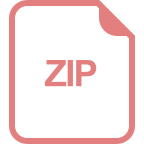
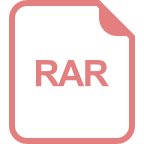
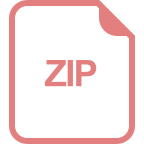
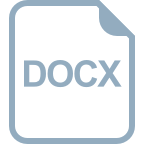