vue3子组件向父组件传递两个子组件渲染的div
时间: 2023-10-01 18:08:40 浏览: 219
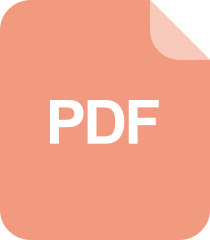
vue 子组件和父组件传值的示例
子组件向父组件传递数据可以通过事件来实现。在子组件中,可以通过 `$emit` 方法触发一个自定义事件,并传递数据作为参数。在父组件中,通过监听这个事件,就可以获取到子组件传递的数据了。
对于你的需求,可以在每个子组件中渲染一个 div,然后在子组件中触发一个自定义事件,将这个 div 作为参数传递给父组件。父组件中监听这个事件,将两个 div 组合起来渲染出来。
下面是一个示例代码:
子组件1:
```html
<template>
<div>
<p>子组件1</p>
<div ref="child1"></div>
</div>
</template>
<script>
export default {
mounted() {
// 在 mounted 钩子中获取 div 元素并传递给父组件
this.$emit('get-child1', this.$refs.child1)
}
}
</script>
```
子组件2:
```html
<template>
<div>
<p>子组件2</p>
<div ref="child2"></div>
</div>
</template>
<script>
export default {
mounted() {
// 在 mounted 钩子中获取 div 元素并传递给父组件
this.$emit('get-child2', this.$refs.child2)
}
}
</script>
```
父组件:
```html
<template>
<div>
<p>父组件</p>
<div ref="parent"></div>
<div ref="child1"></div>
<div ref="child2"></div>
</div>
</template>
<script>
export default {
data() {
return {
child1: null,
child2: null
}
},
mounted() {
// 监听子组件事件,获取子组件传递的 div 元素
this.$on('get-child1', (child1) => {
this.child1 = child1
this.renderDiv()
})
this.$on('get-child2', (child2) => {
this.child2 = child2
this.renderDiv()
})
},
methods: {
renderDiv() {
// 当两个子组件都传递了 div 元素时,将它们组合起来渲染
if (this.child1 && this.child2) {
const parent = this.$refs.parent
parent.appendChild(this.child1)
parent.appendChild(this.child2)
}
}
}
}
</script>
```
阅读全文
相关推荐
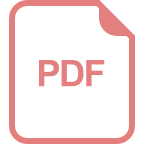
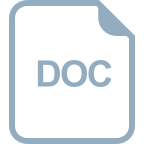




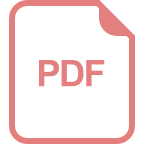
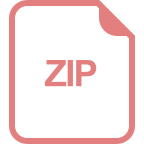
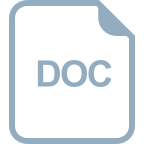
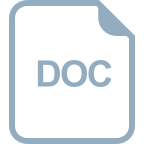
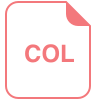
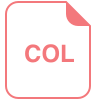
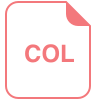



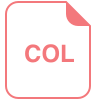
