APU端通过CAN总线输出到汽车仪表端时间信息同步C#
时间: 2023-08-20 20:07:35 浏览: 46
以下是一个使用C#实现APU端通过CAN总线输出到汽车仪表端时间信息同步的简单示例代码:
```csharp
using System;
using System.IO.Ports;
class Program {
static void Main(string[] args) {
// 创建串口实例
SerialPort serialPort = new SerialPort("COM1", 115200, Parity.None, 8, StopBits.One);
// 打开串口
serialPort.Open();
// 定义时间同步帧
byte[] syncFrame = new byte[] { 0x01, 0x02, 0x03, 0x04, 0x05 };
// 同步时间函数
void SyncTime() {
// 获取当前时间
DateTime current = DateTime.Now;
// 发送时间同步帧
serialPort.Write(syncFrame, 0, syncFrame.Length);
// 等待回复帧
byte[] receiveBuffer = new byte[5];
serialPort.Read(receiveBuffer, 0, receiveBuffer.Length);
// 计算同步后的时间
DateTime sync = current.AddSeconds(receiveBuffer[0]);
// 设置系统时间
SystemTime systemTime = new SystemTime();
systemTime.Year = (ushort)sync.Year;
systemTime.Month = (ushort)sync.Month;
systemTime.DayOfWeek = (ushort)sync.DayOfWeek;
systemTime.Day = (ushort)sync.Day;
systemTime.Hour = (ushort)sync.Hour;
systemTime.Minute = (ushort)sync.Minute;
systemTime.Second = (ushort)sync.Second;
Win32.SetSystemTime(ref systemTime);
// 输出同步后的时间
Console.WriteLine("同步后的时间:{0}", sync);
}
// 测试同步时间函数
Console.WriteLine("当前时间:{0}", DateTime.Now);
SyncTime();
// 关闭串口
serialPort.Close();
}
}
// 系统时间结构体
struct SystemTime {
public ushort Year;
public ushort Month;
public ushort DayOfWeek;
public ushort Day;
public ushort Hour;
public ushort Minute;
public ushort Second;
}
// Win32 API
class Win32 {
[System.Runtime.InteropServices.DllImport("kernel32.dll", SetLastError = true)]
public static extern bool SetSystemTime(ref SystemTime st);
}
```
在该示例代码中,我们通过串口将时间同步帧发送到汽车仪表端,并等待其回复帧。然后根据回复帧中的数据计算出同步后的时间,并使用Win32 API设置系统时间。当我们运行该代码时,它会输出当前时间和同步后的时间。需要注意的是,串口的端口号和波特率等参数需要根据实际情况进行配置。
相关推荐
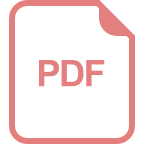
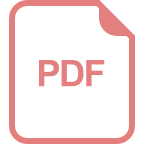
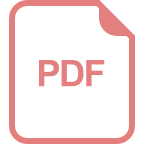














