stl应用
时间: 2023-07-24 12:07:33 浏览: 51
STL(Standard Template Library)是C++语言中的一个标准库,提供了许多数据结构和算法,包括向量、链表、栈、队列、堆、集合、映射、排序、查找等。使用STL可以大大提高程序的效率和可读性,因为它已经封装好了很多常用的数据结构和算法,可以直接拿来使用,而且这些封装好的数据结构和算法都是经过优化的,效率也很高。
下面是几个常用的STL应用示例:
1. 使用向量存储一组数据,并遍历输出:
```c++
#include <vector>
#include <iostream>
int main()
{
std::vector<int> v = {1, 2, 3, 4, 5};
for (auto i : v)
{
std::cout << i << " ";
}
return 0;
}
```
2. 使用集合存储一组数据,并遍历输出:
```c++
#include <set>
#include <iostream>
int main()
{
std::set<int> s = {1, 2, 3, 4, 5};
for (auto i : s)
{
std::cout << i << " ";
}
return 0;
}
```
3. 使用映射存储一组键值对,并遍历输出:
```c++
#include <map>
#include <iostream>
int main()
{
std::map<std::string, int> m = {{"a", 1}, {"b", 2}, {"c", 3}};
for (auto i : m)
{
std::cout << i.first << " " << i.second << std::endl;
}
return 0;
}
```
4. 使用迭代器遍历一个容器:
```c++
#include <vector>
#include <iostream>
int main()
{
std::vector<int> v = {1, 2, 3, 4, 5};
for (auto it = v.begin(); it != v.end(); ++it)
{
std::cout << *it << " ";
}
return 0;
}
```
5. 使用STL算法对容器中的数据进行排序:
```c++
#include <vector>
#include <algorithm>
#include <iostream>
int main()
{
std::vector<int> v = {5, 4, 3, 2, 1};
std::sort(v.begin(), v.end());
for (auto i : v)
{
std::cout << i << " ";
}
return 0;
}
```
相关推荐
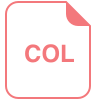
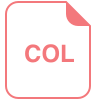
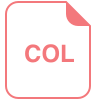
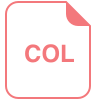
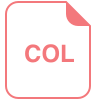









