编辑一个有上传,删除,修改,排序的管理图片的java代码
时间: 2023-11-26 14:03:18 浏览: 89
好的,这是一个简单的图片管理系统,包括上传,删除,修改和排序功能。使用Java语言编写,使用了MySQL数据库存储图片信息。
首先,我们需要创建一个Image类,用于存储每张图片的信息,包括图片ID、图片名称、图片描述和图片路径等。
```java
public class Image {
private int id;
private String name;
private String description;
private String path;
// 构造函数
public Image(int id, String name, String description, String path) {
this.id = id;
this.name = name;
this.description = description;
this.path = path;
}
// getter和setter方法
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public String getPath() {
return path;
}
public void setPath(String path) {
this.path = path;
}
}
```
接下来,我们需要创建一个ImageDao类,用于管理图片的上传、删除、修改和排序等操作。这里我们使用MySQL数据库进行存储和查询。
```java
import java.sql.*;
import java.util.ArrayList;
import java.util.List;
public class ImageDao {
private String url = "jdbc:mysql://localhost:3306/image_db";
private String username = "root";
private String password = "123456";
// 上传图片
public void upload(Image image) {
Connection conn = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
try {
conn = DriverManager.getConnection(url, username, password);
pstmt = conn.prepareStatement("INSERT INTO image VALUES (NULL, ?, ?, ?)");
pstmt.setString(1, image.getName());
pstmt.setString(2, image.getDescription());
pstmt.setString(3, image.getPath());
pstmt.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
if (rs != null) rs.close();
if (pstmt != null) pstmt.close();
if (conn != null) conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
// 删除图片
public void delete(int id) {
Connection conn = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
try {
conn = DriverManager.getConnection(url, username, password);
pstmt = conn.prepareStatement("DELETE FROM image WHERE id = ?");
pstmt.setInt(1, id);
pstmt.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
if (rs != null) rs.close();
if (pstmt != null) pstmt.close();
if (conn != null) conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
// 修改图片
public void update(Image image) {
Connection conn = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
try {
conn = DriverManager.getConnection(url, username, password);
pstmt = conn.prepareStatement("UPDATE image SET name = ?, description = ?, path = ? WHERE id = ?");
pstmt.setString(1, image.getName());
pstmt.setString(2, image.getDescription());
pstmt.setString(3, image.getPath());
pstmt.setInt(4, image.getId());
pstmt.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
if (rs != null) rs.close();
if (pstmt != null) pstmt.close();
if (conn != null) conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
// 按照ID排序图片
public List<Image> sortById() {
List<Image> images = new ArrayList<>();
Connection conn = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
try {
conn = DriverManager.getConnection(url, username, password);
pstmt = conn.prepareStatement("SELECT * FROM image ORDER BY id");
rs = pstmt.executeQuery();
while (rs.next()) {
Image image = new Image(
rs.getInt("id"),
rs.getString("name"),
rs.getString("description"),
rs.getString("path")
);
images.add(image);
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
if (rs != null) rs.close();
if (pstmt != null) pstmt.close();
if (conn != null) conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
return images;
}
}
```
最后,我们可以创建一个测试类,用于测试ImageDao类的各项功能是否正常。
```java
public class Test {
public static void main(String[] args) {
ImageDao dao = new ImageDao();
// 上传图片
Image image1 = new Image(0, "image1", "image1 description", "/path/to/image1.jpg");
dao.upload(image1);
Image image2 = new Image(0, "image2", "image2 description", "/path/to/image2.jpg");
dao.upload(image2);
// 删除图片
dao.delete(1);
// 修改图片
Image image3 = new Image(2, "image3", "image3 description", "/path/to/image3.jpg");
dao.update(image3);
// 按照ID排序图片
List<Image> images = dao.sortById();
for (Image image : images) {
System.out.println(image.getId() + " " + image.getName() + " " + image.getDescription() + " " + image.getPath());
}
}
}
```
阅读全文
相关推荐


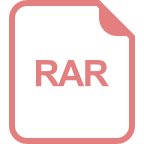


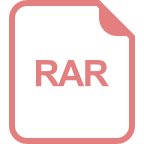

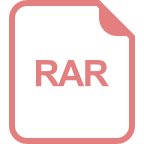







