std::string字符串拼加单引号的示例
时间: 2023-11-15 11:04:32 浏览: 82
好的,我可以为您提供一个示例代码,用于将一个std::string字符串拼接成单引号包裹的形式:
```
#include <string>
#include <iostream>
std::string add_single_quotes(std::string str) {
return "'" + str + "'";
}
int main() {
std::string str = "hello world";
std::string quoted_str = add_single_quotes(str);
std::cout << quoted_str << std::endl; // Output: 'hello world'
return 0;
}
```
这段代码定义了一个名为`add_single_quotes`的函数,它接受一个std::string参数,并返回一个新的std::string,其中原始字符串被用单引号括起来。然后在主函数中,我们将一个字符串传递给该函数并打印结果。
请注意,这只是一个简单的示例,实际应用中您需要考虑更多的字符串处理和错误处理情况。
相关问题
std::string 在c++剔除所有的引号
在 C++ 中,如果你想要从 `std::string` 对象中移除所有引号(单引号 ' 或双引号 "),你可以遍历字符串并检查每个字符。如果遇到引号,就跳过它直到找到对应的另一个引号,然后将它们之间的内容去掉。下面是一个简单的示例:
```cpp
#include <iostream>
#include <string>
std::string remove_quotes(const std::string& str) {
std::string result;
bool inside_quotes = false;
for (char c : str) {
if (c == '\'' || c == '\"') {
inside_quotes = !inside_quotes; // 双重反转标志,因为需要找到对应的引号
continue;
}
if (!inside_quotes) {
result += c; // 如果不在引号内,添加到结果中
}
}
return result;
}
int main() {
std::string input = "Hello, \"world\"! This is a 'test'.";
std::string output = remove_quotes(input);
std::cout << "Output without quotes: " << output << std::endl;
dart std::string c++
在 Dart 中,没有内置的字符串类型,但是可以使用 `String` 类型来表示字符串。相比于 C++ 中的 `std::string`,`String` 类型是一个类,而不是一个结构体,因此使用方法略有不同。
以下是一些基本操作的示例:
1. 声明和初始化
Dart 中的字符串可以使用单引号或双引号括起来,如下所示:
```
String str1 = 'hello';
String str2 = "world";
```
2. 字符串拼接
使用 `+` 运算符来拼接字符串:
```
String str3 = str1 + ' ' + str2; // "hello world"
```
也可以使用 `$` 符号来插入变量:
```
int age = 18;
String str4 = 'I am $age years old.'; // "I am 18 years old."
```
3. 字符串长度
使用 `length` 属性来获取字符串的长度:
```
int len = str3.length; // 11
```
4. 字符串比较
使用 `==` 运算符来判断两个字符串是否相等:
```
String str5 = 'hello world';
bool equal = str3 == str5; // true
```
注意:Dart 中的字符串比较是区分大小写的。
5. 字符串截取
使用 `substring()` 方法来截取字符串:
```
String sub = str3.substring(0, 5); // "hello"
```
以上是一些基本操作的示例,Dart 中的字符串还有很多其他的方法和属性,可以根据实际需求进行使用。
阅读全文
相关推荐
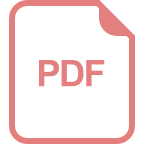
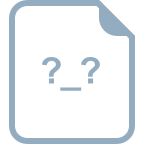
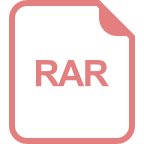
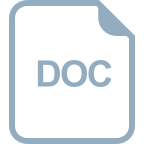
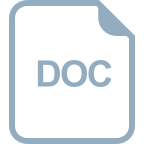
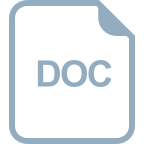
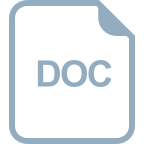
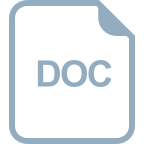
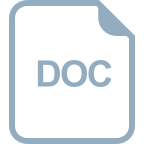
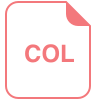
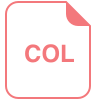
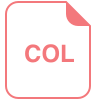
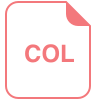
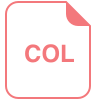
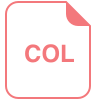
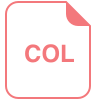
