读入一个存储了3条学生记录的文件file1.dat,将第1条记录和第3条记录交换后保存成文件file2.dat。 学生记录结构如下: struct student { char name[20]; int num; int age; char sex; }; 【输入形式】用c++写代码
时间: 2024-03-02 13:50:20 浏览: 75
以下是用 C++ 写的代码实现上述功能:
```cpp
#include <iostream>
#include <fstream>
#include <cstring>
using namespace std;
struct student {
char name[20];
int num;
int age;
char sex;
};
int main() {
// 读入文件file1.dat
ifstream fin("file1.dat", ios::binary);
if (!fin) {
cout << "Error: cannot open file1.dat" << endl;
return 1;
}
// 获取文件大小
fin.seekg(0, ios::end);
int size = fin.tellg();
fin.seekg(0, ios::beg);
// 计算记录数
int num_records = size / sizeof(student);
// 分配内存并读入数据
student* records = new student[num_records];
fin.read((char*)records, size);
fin.close();
// 交换第1条记录和第3条记录
student tmp = records[0];
records[0] = records[2];
records[2] = tmp;
// 保存到文件file2.dat
ofstream fout("file2.dat", ios::binary);
if (!fout) {
cout << "Error: cannot create file2.dat" << endl;
delete[] records;
return 1;
}
fout.write((char*)records, size);
fout.close();
delete[] records;
cout << "Done!" << endl;
return 0;
}
```
代码的流程如下:
1. 打开文件 file1.dat,读入数据到动态数组 records 中,计算记录数和文件大小。
2. 交换第1条记录和第3条记录。
3. 创建文件 file2.dat,将 records 中的数据写入文件中。
4. 释放内存,关闭文件。
5. 输出完成信息。
注意,该代码中使用了二进制模式进行文件读写,因此在 Windows 平台下需要加上 ios::binary 参数。
阅读全文
相关推荐
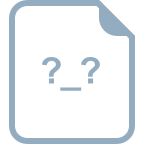

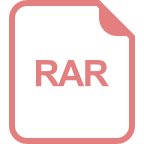
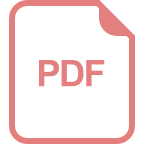
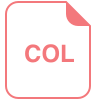
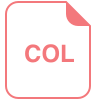
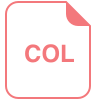
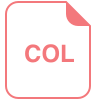
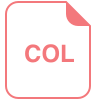
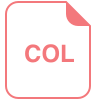
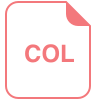
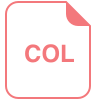
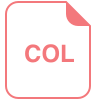
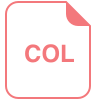
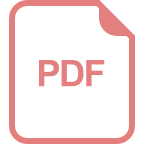