react路由6的goback
时间: 2023-07-19 07:42:43 浏览: 45
在 React Router V6 中,可以使用 `useNavigate` 钩子来管理路由。如果想要回退到上一个页面,可以通过 `useNavigate` 钩子的 `goBack` 方法来实现。例如:
```jsx
import { useNavigate } from 'react-router-dom';
function MyComponent() {
const navigate = useNavigate();
function handleGoBack() {
navigate(-1);
}
return (
<div>
<button onClick={handleGoBack}>返回上一页</button>
</div>
);
}
```
在上述代码中,我们通过 `useNavigate` 钩子创建了 `navigate` 对象,并在 `handleGoBack` 函数中调用了 `navigate(-1)` 方法来回退到上一个页面。
相关问题
React 路由跳转
React中有几种方式实现页面跳转。第一种是使用react-router-dom中的Link组件,通过在Link组件中设置to属性来指定跳转的路径。可以在to属性中设置pathname为目标路径,state为要传递的数据。这种方式适用于点击按钮或其他组件进行页面跳转。
第二种方式是使用react-router-redux中的push方法进行页面跳转。通过在dispatch中调用push方法并传入目标路径和要传递的数据,可以实现页面跳转。可以使用push方法跳转到指定路径,或者使用replace方法替换历史记录中的当前位置。还可以使用go方法在历史记录中向前或向后移动相对数量的位置,或者使用goForward方法向前移动一个位置,使用goBack方法向后移动一个位置。
第三种方式是使用RouteComponentProps中的history进行页面回退。通过在组件的props中引入history对象,可以使用history.goBack()方法实现页面回退。这种方式适用于需要在页面中触发回退操作的场景。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
react路由重定向1
在使用React Router进行重定向时,有几种方法可以实现。首先,你可以使用BrowserRouter来引入重定向所需的组件,比如Redirect。在代码中,你可以使用<Redirect to='路由路径'></Redirect>来实现动态跳转。另外,你也可以通过this.props.history.push('路由路径',{参数键值对})来进行跳转,并通过this.props.location.state.键名获取参数。除此之外,你还可以使用this.props.history.go(数值)来根据栈来进行跳转,使用this.props.history.goBack()来返回上一页,使用this.props.history.goForward()来前进到下一页。这些方法都可以帮助你实现React路由的重定向功能。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* [react 路由重定向、动态跳转](https://blog.csdn.net/weixin_43294560/article/details/104493912)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 33.333333333333336%"]
- *2* [使用react-router4.0实现重定向和404功能的方法](https://download.csdn.net/download/weixin_38545463/12774142)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 33.333333333333336%"]
- *3* [2.react路由重定向](https://blog.csdn.net/weixin_45414972/article/details/131210334)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 33.333333333333336%"]
[ .reference_list ]
相关推荐
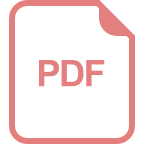
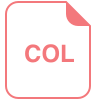
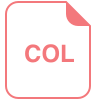
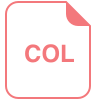
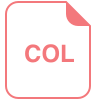








