用C语言做一个教材购销系统
时间: 2024-01-12 17:02:34 浏览: 275
要实现一个教材购销系统,需要考虑以下几个方面:
1. 数据库设计:需要设计一个数据库来存储教材的信息,包括名称、作者、出版社、价格等等。
2. 用户界面设计:需要设计一个用户界面,让用户可以浏览教材信息、查询教材、购买教材等等。
3. 购买流程:需要设计一个购买流程,让用户可以选择购买的教材、输入购买数量、选择支付方式等等。
以下是一个用C语言实现基本功能的伪代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
//定义教材结构体
typedef struct {
char name[50];
char author[50];
char publisher[50];
float price;
int quantity;
} Book;
//定义教材数组
Book books[100];
//定义教材数量
int num_books = 0;
//添加教材
void add_book() {
Book book;
printf("请输入教材名称:");
scanf("%s", book.name);
printf("请输入教材作者:");
scanf("%s", book.author);
printf("请输入教材出版社:");
scanf("%s", book.publisher);
printf("请输入教材价格:");
scanf("%f", &book.price);
printf("请输入教材数量:");
scanf("%d", &book.quantity);
books[num_books++] = book;
}
//浏览教材
void view_books() {
printf("教材名称\t教材作者\t教材出版社\t教材价格\t教材数量\n");
for (int i = 0; i < num_books; i++) {
printf("%s\t%s\t%s\t%.2f\t%d\n", books[i].name, books[i].author, books[i].publisher, books[i].price, books[i].quantity);
}
}
//查询教材
void search_book() {
char name[50];
printf("请输入教材名称:");
scanf("%s", name);
for (int i = 0; i < num_books; i++) {
if (strcmp(books[i].name, name) == 0) {
printf("教材名称\t教材作者\t教材出版社\t教材价格\t教材数量\n");
printf("%s\t%s\t%s\t%.2f\t%d\n", books[i].name, books[i].author, books[i].publisher, books[i].price, books[i].quantity);
return;
}
}
printf("没有找到该教材!\n");
}
//购买教材
void buy_book() {
char name[50];
int quantity;
printf("请输入教材名称:");
scanf("%s", name);
for (int i = 0; i < num_books; i++) {
if (strcmp(books[i].name, name) == 0) {
printf("请输入购买数量:");
scanf("%d", &quantity);
if (quantity > books[i].quantity) {
printf("库存不足!\n");
return;
}
printf("请选择支付方式(1.支付宝,2.微信):");
int choice;
scanf("%d", &choice);
if (choice == 1) {
printf("支付宝支付成功!\n");
} else if (choice == 2) {
printf("微信支付成功!\n");
} else {
printf("无效的支付方式!\n");
}
books[i].quantity -= quantity;
printf("购买成功!\n");
return;
}
}
printf("没有找到该教材!\n");
}
int main() {
int choice;
while (1) {
printf("请选择操作:\n");
printf("1.添加教材\n");
printf("2.浏览教材\n");
printf("3.查询教材\n");
printf("4.购买教材\n");
printf("5.退出\n");
printf("请输入选项:");
scanf("%d", &choice);
switch (choice) {
case 1:
add_book();
break;
case 2:
view_books();
break;
case 3:
search_book();
break;
case 4:
buy_book();
break;
case 5:
return 0;
default:
printf("无效的选项!\n");
}
}
}
```
以上是一个基本教材购销系统的实现,代码中还有很多需要完善的地方,比如数据库的实现、用户界面的美化等等。
阅读全文
相关推荐
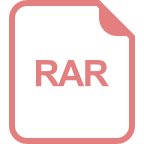
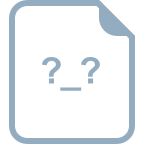
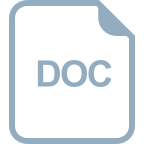
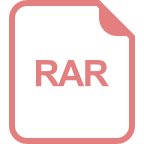
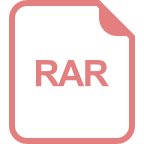
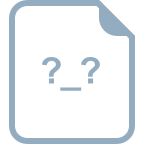
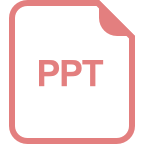
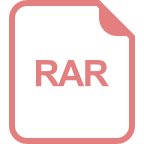
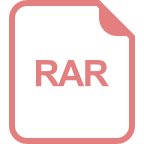