android studio读取sdcard download 文件夹下图片
时间: 2024-12-08 12:25:00 浏览: 15
在Android Studio中,如果你想从SD卡下载文件夹里读取图片,你需要遵守Android的安全性和权限规则,并确保应用已经获得了读取外部存储的权限。以下是大致步骤:
1. **添加权限**:
在`AndroidManifest.xml`中添加读取外部存储的权限:
```xml
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" />
```
如果目标API级别较低(23及以下),还需要添加运行时请求权限。
2. **检查权限**:
使用`ContextCompat.checkSelfPermission()`检查权限是否已授予,如果未授予,则需要请求:
```java
if (ActivityCompat.checkSelfPermission(this, Manifest.permission.READ_EXTERNAL_STORAGE)
!= PackageManager.PERMISSION_GRANTED) {
// 请求权限
}
```
3. **访问文件路径**:
获取SD卡的路径通常是通过Environment类,然后指定`DOWNLOADS`目录:
```java
String externalStoragePath = Environment.getExternalStorageDirectory().getPath();
String downloadsPath = externalStoragePath + File.separator + "Download";
```
4. **读取图片**:
有了路径之后,你可以使用`File`类和` BitmapFactory`来读取图片:
```java
File imageFile = new File(downloadsPath, "image.jpg");
try {
BitmapFactory.Options options = new BitmapFactory.Options();
options.inJustDecodeBounds = true; // 只获取尺寸信息,不用加载数据
BitmapFactory.decodeFile(imageFile.getAbsolutePath(), options);
int width = options.outWidth;
int height = options.outHeight;
options.inJustDecodeBounds = false; // 现在可以加载数据
Bitmap bitmap = BitmapFactory.decodeFile(imageFile.getAbsolutePath(), options);
} catch (IOException e) {
e.printStackTrace();
}
```
5. **处理异常**:
要确保处理可能出现的文件不存在、权限错误等异常情况。
记得在实际项目中处理好异常,并妥善关闭资源,如Bitmap对象。
阅读全文
相关推荐
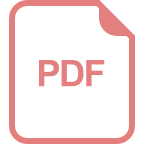
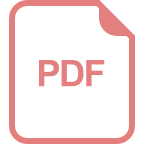
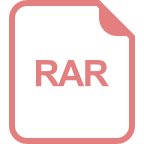
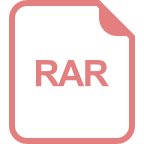
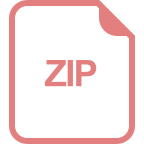
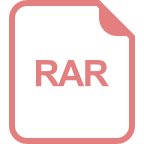
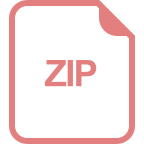
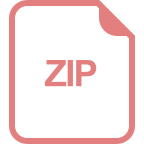
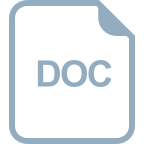
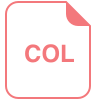
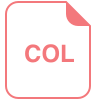
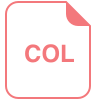
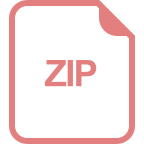
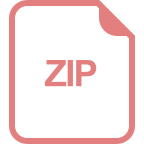
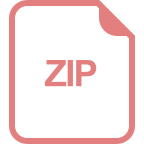
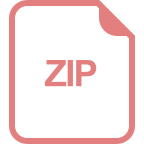
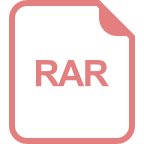
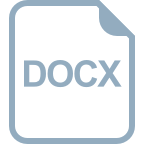