请生成上一个问题的代码
时间: 2024-02-09 12:09:25 浏览: 78
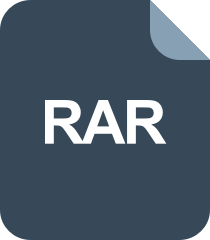
问题的代码
好的,这里给出一个简单的实现,仅供参考:
1. res/layout/activity_register.xml (注册页面布局文件)
```xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<EditText
android:id="@+id/edit_account"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="账号" />
<EditText
android:id="@+id/edit_password"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="密码"
android:inputType="textPassword" />
<Button
android:id="@+id/btn_register"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="注册" />
</LinearLayout>
```
2. res/layout/activity_login.xml (登录页面布局文件)
```xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<EditText
android:id="@+id/edit_account"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="账号" />
<EditText
android:id="@+id/edit_password"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="密码"
android:inputType="textPassword" />
<CheckBox
android:id="@+id/check_remember"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="记住密码" />
<Button
android:id="@+id/btn_login"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="登录" />
</LinearLayout>
```
3. RegisterActivity.java (注册页面的Activity)
```java
public class RegisterActivity extends AppCompatActivity {
private EditText mEditAccount;
private EditText mEditPassword;
private Button mBtnRegister;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_register);
mEditAccount = findViewById(R.id.edit_account);
mEditPassword = findViewById(R.id.edit_password);
mBtnRegister = findViewById(R.id.btn_register);
mBtnRegister.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String account = mEditAccount.getText().toString().trim();
String password = mEditPassword.getText().toString().trim();
SharedPreferences sp = getSharedPreferences("user", MODE_PRIVATE);
if (sp.contains(account)) {
Toast.makeText(RegisterActivity.this, "账号已存在", Toast.LENGTH_SHORT).show();
} else {
SharedPreferences.Editor editor = sp.edit();
editor.putString(account, password);
editor.apply();
Toast.makeText(RegisterActivity.this, "注册成功", Toast.LENGTH_SHORT).show();
finish();
}
}
});
}
}
```
4. LoginActivity.java (登录页面的Activity)
```java
public class LoginActivity extends AppCompatActivity {
private EditText mEditAccount;
private EditText mEditPassword;
private CheckBox mCheckRemember;
private Button mBtnLogin;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_login);
mEditAccount = findViewById(R.id.edit_account);
mEditPassword = findViewById(R.id.edit_password);
mCheckRemember = findViewById(R.id.check_remember);
mBtnLogin = findViewById(R.id.btn_login);
mBtnLogin.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String account = mEditAccount.getText().toString().trim();
String password = mEditPassword.getText().toString().trim();
SharedPreferences sp = getSharedPreferences("user", MODE_PRIVATE);
if (sp.contains(account) && sp.getString(account, "").equals(password)) {
Toast.makeText(LoginActivity.this, "登录成功", Toast.LENGTH_SHORT).show();
if (mCheckRemember.isChecked()) {
SharedPreferences.Editor editor = sp.edit();
editor.putString("last_account", account);
editor.putString("last_password", password);
editor.apply();
}
finish();
} else {
Toast.makeText(LoginActivity.this, "登录失败", Toast.LENGTH_SHORT).show();
}
}
});
SharedPreferences sp = getSharedPreferences("user", MODE_PRIVATE);
String lastAccount = sp.getString("last_account", "");
String lastPassword = sp.getString("last_password", "");
if (!TextUtils.isEmpty(lastAccount) && !TextUtils.isEmpty(lastPassword)) {
mEditAccount.setText(lastAccount);
mEditPassword.setText(lastPassword);
mCheckRemember.setChecked(true);
}
}
}
```
这个实现方式是基于SharedPreferences来保存注册的账号和密码信息,并在登录页面中进行匹配,实现注册登录功能。如果需要更高级的功能,例如加密存储用户密码等,可以参考更多的Android开发教程。
阅读全文
相关推荐
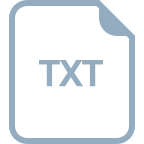
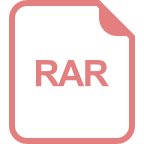
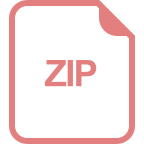
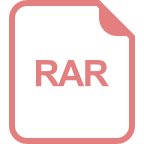
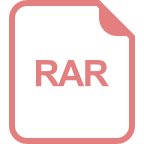
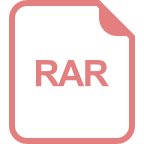
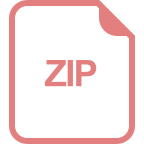
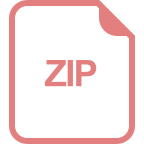
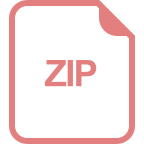
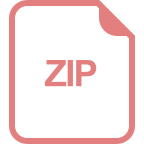
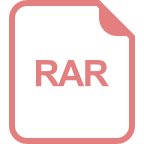
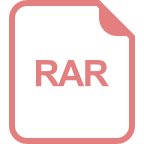
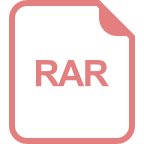
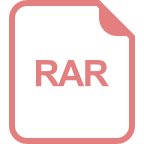