import requests from bs4 import BeautifulSoup from pprint import pprint for page in range(5): url = "https://movie.douban.com/subject/30391186/comments?start={}&limit=20&sort=new_score&status=P".format(page*20) headers = { "User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3" } response = requests.get(url, headers=headers) soup = BeautifulSoup(response.text, "html.parser") comments = [] for comment in soup.select(".comment-item"): content = comment.select_one(".comment-content").get_text().strip() author = comment.select_one(".comment-info a").get_text().strip() likes = comment.select_one(".votes").get_text().strip() time = comment.select_one(".comment-time").get_text().strip() location = comment.select_one(".comment-location").contents[-1].strip() comments.append({ "content": content, "author": author, "likes": likes, "time": time, "location": location }) print("第{}页的数据:".format(page+1)) pprint(comments),我要将数据存入mysql
时间: 2024-04-03 18:35:01 浏览: 29
非常感谢您的问题。根据您提供的代码,您已经成功获取了豆瓣电影《误杀》的评论数据,并将其存储在了`comments`变量中。如果您想将这些数据存储到MySQL数据库中,您可以按照以下步骤进行操作:
1. 安装MySQL驱动程序
首先,您需要安装MySQL数据库的Python驱动程序。您可以使用以下命令在命令行中安装:
```
pip install mysql-connector-python
```
2. 连接到MySQL数据库
接下来,您需要使用Python的`mysql.connector`模块连接到MySQL数据库。您需要提供MySQL数据库的主机名、用户名、密码和数据库名称。以下是一个示例代码:
```python
import mysql.connector
cnx = mysql.connector.connect(user='username', password='password',
host='localhost', database='database_name')
```
3. 创建数据表
在将数据存储到MySQL数据库之前,您需要先创建一个数据表,用于存储评论数据。您可以使用以下命令在MySQL数据库中创建一个名为`comments`的数据表:
```sql
CREATE TABLE comments (
id INT NOT NULL AUTO_INCREMENT PRIMARY KEY,
content TEXT,
author VARCHAR(255),
likes INT,
time VARCHAR(255),
location VARCHAR(255)
);
```
这个数据表包括五个字段:`id`、`content`、`author`、`likes`、`time`和`location`。其中,`id`字段为自增长主键,用于唯一标识每条评论数据。
4. 将数据插入到数据表中
现在,您已经连接到MySQL数据库并创建了一个数据表,可以将评论数据插入到数据表中。您可以使用以下代码将`comments`列表中的每个元素插入到MySQL数据库中:
```python
cursor = cnx.cursor()
for comment in comments:
content = comment["content"].replace("'", "\\'")
author = comment["author"].replace("'", "\\'")
likes = int(comment["likes"])
time = comment["time"].replace("'", "\\'")
location = comment["location"].replace("'", "\\'")
sql = "INSERT INTO comments (content, author, likes, time, location) VALUES ('{}', '{}', {}, '{}', '{}')".format(
content, author, likes, time, location)
cursor.execute(sql)
cnx.commit()
```
在这个代码中,您需要注意将评论数据中的单引号转义,以避免插入到MySQL数据库中时出现语法错误。此外,您还需要使用`cnx.commit()`函数提交更改,以确保数据被保存到MySQL数据库中。
5. 关闭数据库连接
最后,您需要使用以下代码关闭MySQL数据库连接:
```python
cursor.close()
cnx.close()
```
这样,您就可以将评论数据存储到MySQL数据库中了。希望这个回答能对您有所帮助。
相关推荐
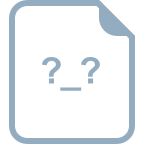
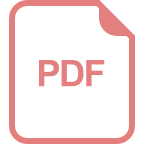
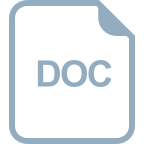












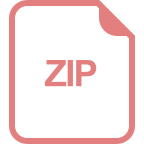
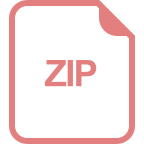
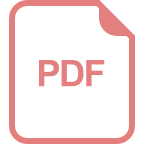