从文本文件读取学生信息,并存储到另一个文件。C++C++
时间: 2023-12-28 07:04:34 浏览: 88
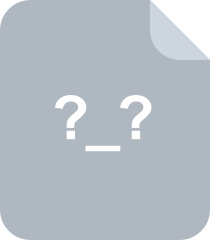
C++实现学生信息用文件存储
下面是一个简单的 C++ 代码示例,可以从文本文件中读取学生信息,并将其存储到另一个文件中:
```c++
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
struct Student {
string name;
int age;
double score;
};
int main() {
ifstream infile("input.txt"); // 打开输入文件
ofstream outfile("output.txt"); // 打开输出文件
if (!infile) {
cerr << "Error: cannot open input file." << endl;
return -1;
}
if (!outfile) {
cerr << "Error: cannot open output file." << endl;
return -1;
}
Student stu;
while (infile >> stu.name >> stu.age >> stu.score) { // 从输入文件中读取学生信息
outfile << stu.name << "\t" << stu.age << "\t" << stu.score << endl; // 将学生信息写入输出文件
}
infile.close(); // 关闭输入文件
outfile.close(); // 关闭输出文件
return 0;
}
```
在上面的示例代码中,我们使用了 `ifstream` 和 `ofstream` 类来分别打开输入文件和输出文件。然后,我们定义了一个 `Student` 结构体来存储学生信息。在主函数中,我们使用 `while` 循环来从输入文件中读取每个学生的信息,并将其存储到 `Student` 结构体中。最后,我们使用 `ofstream` 类将学生信息写入输出文件中。最后,我们关闭了输入文件和输出文件。
请注意,这只是一个简单的示例,您可以根据自己的需求进行修改和扩展。
阅读全文
相关推荐
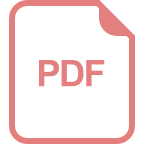
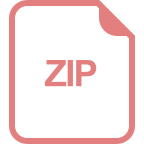
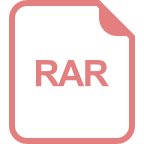
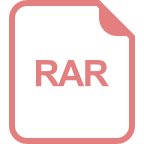
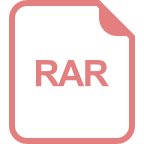
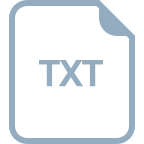
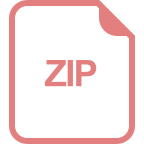
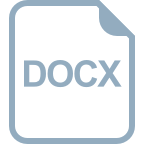
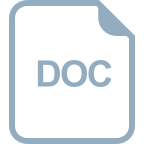
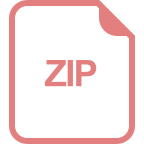
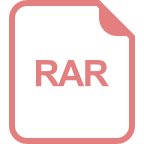
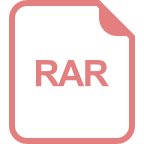
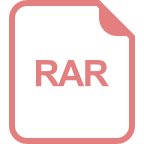
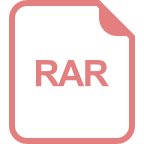
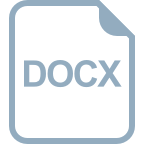
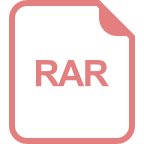