7-1 单向链表2 分数 25 作者 wangxiu 单位 福州大学 编程实现:输入一个正整数 repeat (0<repeat<10),做 repeat 次下列运算: 输入一个正整数 n(0<n<=9)和一组(n个)整数,建立一个单向链表,再输入一个整数 x,将链表中最后一个与x相等的整数删除。 输入输出示例:括号内为说明 输入样例: 3 (repeat=3) 5 (数据的个数n=5) 1 2 4 3 7 (5个整数) 4 (待删除整数x=4) 5 (数据的个数n=5) 2 1 5 7 5 (5个整数) 5 (待删除整数x=5) 3 (数据的个数n=3) 1 2 4 (3个整数) 100 (待删除整数x=100) 输出样例: size=4:1 2 3 7 size=4:2 1 5 7 size=3:1 2 4
时间: 2024-03-26 19:38:32 浏览: 16
下面是符合要求的 C++ 代码:
```c++
#include <iostream>
using namespace std;
struct Node {
int val;
Node* next;
Node(int x) : val(x), next(NULL) {}
};
Node* createList(int n) { // 创建链表
Node* head = new Node(0);
Node* cur = head;
for (int i = 0; i < n; ++i) {
int x;
cin >> x;
Node* node = new Node(x);
cur->next = node;
cur = node;
}
return head->next;
}
Node* deleteNode(Node* head, int x) { // 删除节点
Node* dummy = new Node(0);
dummy->next = head;
Node* prev = dummy;
Node* cur = head;
while (cur) {
if (cur->val == x) {
prev->next = cur->next;
delete cur;
return dummy->next;
}
prev = cur;
cur = cur->next;
}
return dummy->next;
}
void printList(Node* head) { // 输出链表
int cnt = 0;
while (head) {
if (cnt > 0) cout << " ";
cout << head->val;
head = head->next;
++cnt;
}
cout << endl;
cout << "size=" << cnt << ":";
}
int main() {
int repeat;
cin >> repeat;
while (repeat--) {
int n, x;
cin >> n;
Node* head = createList(n);
cin >> x;
head = deleteNode(head, x);
printList(head);
}
return 0;
}
```
这个程序先读入 repeat,表示有 repeat 组数据需要处理。每组数据首先读入 n,然后调用 createList 函数创建链表。接着读入 x,调用 deleteNode 函数删除链表中最后一个与 x 相等的节点。最后调用 printList 函数输出链表。
相关推荐
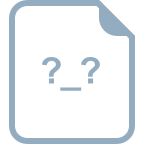











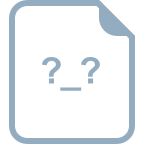