用代码实现linux系统中,线程之间的通信。c语言实现
时间: 2024-03-02 12:27:03 浏览: 57
在 Linux 系统中,线程之间的通信可以使用多种机制,例如共享内存、消息队列、信号量等。以下是使用共享内存实现线程之间通信的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <pthread.h>
#include <sys/mman.h>
#define SHM_SIZE 1024
void *producer(void *arg);
void *consumer(void *arg);
int main()
{
int *shm_ptr;
pthread_t tid1, tid2;
// 创建共享内存
int shm_fd = shm_open("/my_shm", O_CREAT | O_RDWR, 0666);
if (shm_fd == -1) {
perror("shm_open");
exit(EXIT_FAILURE);
}
if (ftruncate(shm_fd, SHM_SIZE) == -1) {
perror("ftruncate");
exit(EXIT_FAILURE);
}
shm_ptr = mmap(NULL, SHM_SIZE, PROT_READ | PROT_WRITE, MAP_SHARED, shm_fd, 0);
if (shm_ptr == MAP_FAILED) {
perror("mmap");
exit(EXIT_FAILURE);
}
// 初始化共享内存
*shm_ptr = 0;
// 创建生产者和消费者线程
pthread_create(&tid1, NULL, producer, shm_ptr);
pthread_create(&tid2, NULL, consumer, shm_ptr);
// 等待线程结束
pthread_join(tid1, NULL);
pthread_join(tid2, NULL);
// 销毁共享内存
munmap(shm_ptr, SHM_SIZE);
shm_unlink("/my_shm");
return 0;
}
void *producer(void *arg)
{
int *shm_ptr = (int *)arg;
for (int i = 0; i < 10; i++) {
(*shm_ptr)++;
printf("Producer: %d\n", *shm_ptr);
sleep(1);
}
pthread_exit(NULL);
}
void *consumer(void *arg)
{
int *shm_ptr = (int *)arg;
for (int i = 0; i < 10; i++) {
printf("Consumer: %d\n", *shm_ptr);
sleep(1);
}
pthread_exit(NULL);
}
```
在上面的代码中,我们使用 `shm_open` 函数创建了一个共享内存对象,然后使用 `mmap` 函数将其映射到当前进程的地址空间中。生产者线程通过修改共享内存中的值来向消费者线程传递数据。注意,由于线程共享同一块内存区域,因此需要使用同步机制来避免竞争条件。
阅读全文
相关推荐
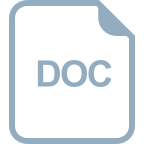
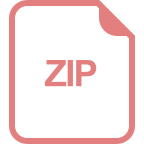
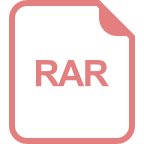
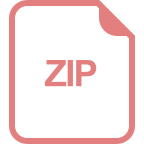
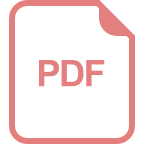
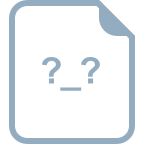
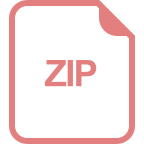
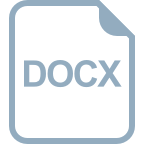
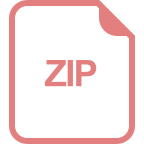
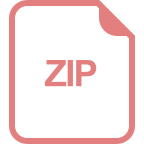
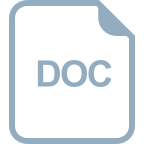
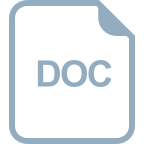
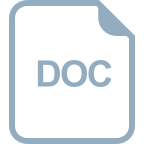
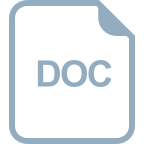
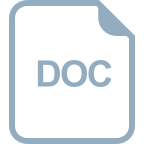
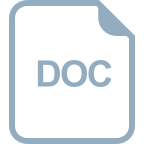