用java写一个带有图形界面的简易ATM自动提款机
时间: 2023-12-09 18:07:04 浏览: 147
好的,我可以为您提供一个简单的 Java ATM 提款机图形界面代码示例。这个示例包括账户验证、余额查询、取款以及退出功能。请注意,这只是一个简单的示例,并不包含所有的功能和安全措施。
```
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class ATMGUI extends JFrame implements ActionListener {
private JLabel usernameLabel;
private JLabel passwordLabel;
private JTextField usernameField;
private JPasswordField passwordField;
private JButton loginButton;
private JButton balanceButton;
private JButton withdrawButton;
private JButton exitButton;
private JLabel balanceLabel;
private JTextField amountField;
private JLabel messageLabel;
private String userName = "John"; // 用户名
private String password = "1234"; // 密码
private double balance = 1000; // 账户余额
public ATMGUI() {
super("ATM 自动提款机");
// 创建组件
usernameLabel = new JLabel("用户名:");
passwordLabel = new JLabel("密码:");
usernameField = new JTextField(10);
passwordField = new JPasswordField(10);
loginButton = new JButton("登陆");
balanceButton = new JButton("查询余额");
withdrawButton = new JButton("取款");
exitButton = new JButton("退出");
balanceLabel = new JLabel("账户余额为:" + balance);
amountField = new JTextField(10);
messageLabel = new JLabel("");
// 设置布局
setLayout(new GridLayout(5, 2, 5, 5));
// 添加组件
add(usernameLabel);
add(usernameField);
add(passwordLabel);
add(passwordField);
add(loginButton);
add(new JLabel(""));
add(balanceButton);
add(withdrawButton);
add(amountField);
add(exitButton);
// 注册事件监听器
loginButton.addActionListener(this);
balanceButton.addActionListener(this);
withdrawButton.addActionListener(this);
exitButton.addActionListener(this);
// 设置窗口属性
setSize(400, 200);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLocationRelativeTo(null);
setVisible(true);
}
@Override
public void actionPerformed(ActionEvent e) {
if (e.getSource() == loginButton) {
String inputUserName = usernameField.getText();
String inputPassword = new String(passwordField.getPassword());
if (userName.equals(inputUserName) && password.equals(inputPassword)) {
messageLabel.setText("登陆成功!");
add(messageLabel);
} else {
messageLabel.setText("用户名或密码错误,请重新输入!");
add(messageLabel);
}
} else if (e.getSource() == balanceButton) {
messageLabel.setText("账户余额为:" + balance);
add(messageLabel);
} else if (e.getSource() == withdrawButton) {
double amount = Double.parseDouble(amountField.getText());
if (amount > balance) {
messageLabel.setText("余额不足!");
add(messageLabel);
} else {
balance -= amount;
messageLabel.setText("取款成功!账户余额为:" + balance);
add(messageLabel);
}
} else if (e.getSource() == exitButton) {
System.exit(0);
}
}
public static void main(String[] args) {
new ATMGUI();
}
}
```
这是一个带有图形界面的简易 ATM 自动提款机的 Java 代码示例。希望能够帮到您!
阅读全文
相关推荐
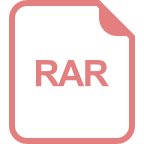
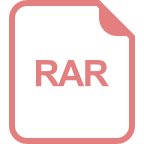
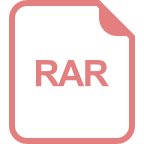
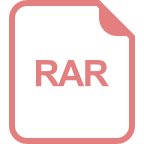
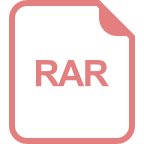
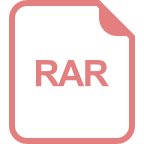
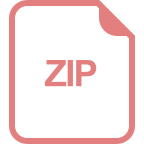




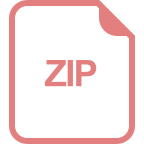
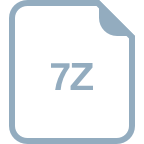
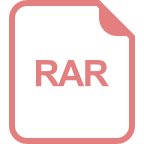
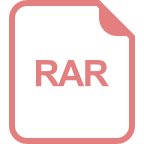
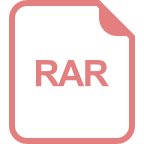
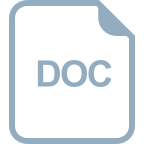
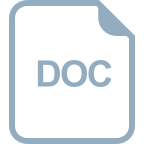