将上述代码中的中文全部用英文代替
时间: 2024-02-09 16:11:07 浏览: 51
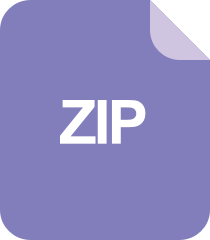
中文转英文代码
Here is the optimized code that allows the user to input an integer array, moves all zeros to the end of the array while maintaining the relative order of non-zero elements:
```c
#include <stdio.h>
#include <stdlib.h>
void moveZeros(int* nums, int numsSize) {
int i, j = 0;
for (i = 0; i < numsSize; i++) {
if (nums[i] != 0) {
nums[j++] = nums[i];
}
}
while (j < numsSize) {
nums[j++] = 0;
}
}
int main() {
int *nums, numsSize, i;
printf("Please enter the length of the integer array: ");
scanf("%d", &numsSize);
nums = (int*)malloc(numsSize * sizeof(int));
printf("Please enter the integer array: ");
for (i = 0; i < numsSize; i++) {
scanf("%d", &nums[i]);
}
moveZeros(nums, numsSize);
printf("The modified array is: ");
for (i = 0; i < numsSize; i++) {
printf("%d ", nums[i]);
}
free(nums);
return 0;
}
```
In this optimized code, we used dynamic memory allocation to allocate memory for the integer array based on the user's input, rather than defining a fixed size for the array. We used `malloc` to allocate memory and `free` to release it at the end of the program. The program allows the user to input an integer array, calls the `moveZeros` function to move all the zeros to the end of the array while maintaining the relative order of non-zero elements, and prints out the modified array.
阅读全文
相关推荐
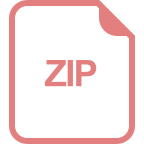
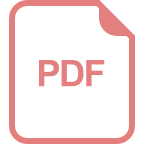
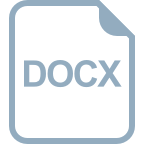
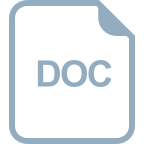

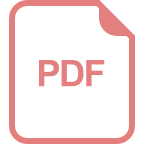
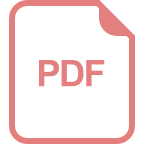
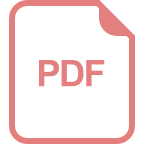
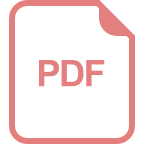
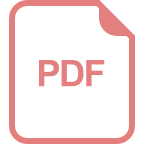
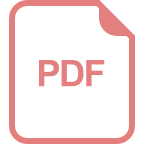
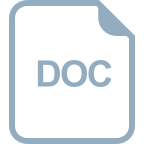
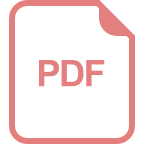
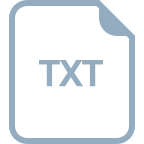
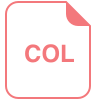
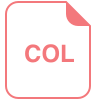
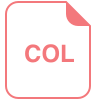