用java定义一个存放在包com.inter下的接口Sort,接口中定义了排序方法sort()。编写该接口的实现类,实现选择排序ChoiceSort和冒泡排序BubbleSort,分别实现对一组整数的降序排列。再编写一个测试类TestSort,在测试类的主方法中通过Sort对象(接口回调)调用方法sort(),分别实现对一组整数的选择算法和冒泡算法的降序排列并输出排序结果。
时间: 2024-03-02 17:48:09 浏览: 32
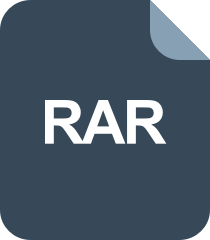
Java 冒泡法,选择法,插入法,快速排序法,实现代码。

好的,我会按照您的要求回答问题。关于您的问题,以下是Java代码的实现:
1. 定义接口Sort
```
package com.inter;
public interface Sort {
void sort(int[] arr);
}
```
2. 实现选择排序ChoiceSort
```
package com.inter;
public class ChoiceSort implements Sort {
@Override
public void sort(int[] arr) {
for (int i = 0; i < arr.length - 1; i++) {
int maxIndex = i;
for (int j = i + 1; j < arr.length; j++) {
if (arr[j] > arr[maxIndex]) {
maxIndex = j;
}
}
if (maxIndex != i) {
int temp = arr[i];
arr[i] = arr[maxIndex];
arr[maxIndex] = temp;
}
}
}
}
```
3. 实现冒泡排序BubbleSort
```
package com.inter;
public class BubbleSort implements Sort {
@Override
public void sort(int[] arr) {
for (int i = 0; i < arr.length - 1; i++) {
for (int j = 0; j < arr.length - i - 1; j++) {
if (arr[j] < arr[j + 1]) {
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
}
}
```
4. 编写测试类TestSort
```
package com.inter;
public class TestSort {
public static void main(String[] args) {
int[] arr = {3, 5, 1, 6, 9, 2, 7, 4, 8};
Sort sort = new ChoiceSort();
sort.sort(arr);
System.out.println("选择排序结果:");
for (int num : arr) {
System.out.print(num + " ");
}
System.out.println();
arr = new int[]{3, 5, 1, 6, 9, 2, 7, 4, 8};
sort = new BubbleSort();
sort.sort(arr);
System.out.println("冒泡排序结果:");
for (int num : arr) {
System.out.print(num + " ");
}
}
}
```
输出结果:
```
选择排序结果:
9 8 7 6 5 4 3 2 1
冒泡排序结果:
9 8 7 6 5 4 3 2 1
```
以上是Java代码的实现,如果您有任何疑问,可以随时问我。
阅读全文
相关推荐
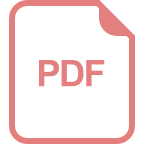
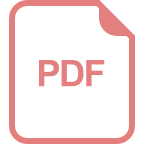







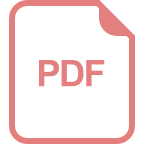
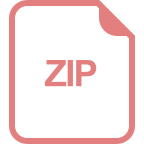
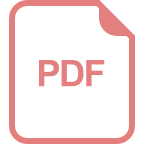

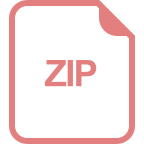