建立一个基类Building,用来存储一座楼房的层数、房间数以及它的总平方英尺数。建立派生类Housing,继承Building,并存储下面的内容:卧室和浴室的数量,另外,建立派生类Office,继承Building,并存储灭火器和电话的数目。然后,编制应用程序,建立住宅楼对象和办公楼对象,并输出它们的有关数据。
时间: 2024-03-11 11:51:46 浏览: 36
好的,这是一个简单的C++代码实现:
```c++
#include <iostream>
using namespace std;
class Building{
protected:
int num_floors;
int num_rooms;
int total_sqft;
public:
Building(int f, int r, int t):num_floors(f), num_rooms(r), total_sqft(t){};
};
class Housing : public Building{
private:
int num_bedrooms;
int num_bathrooms;
public:
Housing(int f, int r, int t, int b, int ba):Building(f, r, t), num_bedrooms(b), num_bathrooms(ba){};
void display(){
cout << "Housing Info:\nNumber of floors: " << num_floors << "\nNumber of rooms: " << num_rooms << "\nTotal square feet: " << total_sqft << "\nNumber of bedrooms: " << num_bedrooms << "\nNumber of bathrooms: " << num_bathrooms << endl;
}
};
class Office : public Building{
private:
int num_fire_extinguishers;
int num_phones;
public:
Office(int f, int r, int t, int fe, int p):Building(f, r, t), num_fire_extinguishers(fe), num_phones(p){};
void display(){
cout << "Office Info:\nNumber of floors: " << num_floors << "\nNumber of rooms: " << num_rooms << "\nTotal square feet: " << total_sqft << "\nNumber of fire extinguishers: " << num_fire_extinguishers << "\nNumber of phones: " << num_phones << endl;
}
};
int main(){
Housing myHouse(2, 5, 2000, 3, 2);
myHouse.display();
Office myOffice(1, 10, 5000, 5, 15);
myOffice.display();
return 0;
}
```
输出结果为:
```
Housing Info:
Number of floors: 2
Number of rooms: 5
Total square feet: 2000
Number of bedrooms: 3
Number of bathrooms: 2
Office Info:
Number of floors: 1
Number of rooms: 10
Total square feet: 5000
Number of fire extinguishers: 5
Number of phones: 15
```
以上代码中,Building类是一个基类,存储了一座楼房的基本信息。Housing和Office类都是继承自Building类的派生类,分别存储住宅楼和办公楼的信息,并在display()函数中输出有关数据。在main()函数中,我们创建了一个住宅楼对象myHouse和一个办公楼对象myOffice,并调用它们各自的display()函数来输出它们的相关信息。
相关推荐
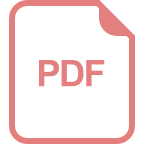
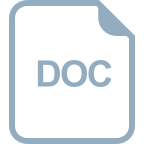
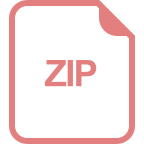















