用Python写一个自动剪辑的代码
时间: 2024-04-25 21:03:23 浏览: 207
这个需求可能比较复杂,需要涉及到音视频处理和算法设计。以下是一个基本的思路和示例代码,仅供参考:
1. 导入必要的库
```python
import moviepy.editor as mp
import numpy as np
```
2. 加载视频文件
```python
clip = mp.VideoFileClip("video.mp4")
```
3. 提取音频信号
```python
audio = clip.audio.to_soundarray()
```
4. 计算音频信号的能量
```python
energy = np.sum(audio**2, axis=1)
```
5. 设定阈值并寻找连续段
```python
threshold = 10000
segments = []
segment_start = None
for i, e in enumerate(energy):
if e > threshold and segment_start is None:
segment_start = i
elif e < threshold and segment_start is not None:
segments.append((segment_start, i))
segment_start = None
if segment_start is not None:
segments.append((segment_start, len(energy)))
```
6. 根据找到的连续段裁剪视频
```python
clips = []
for start, end in segments:
subclip = clip.subclip(start/clip.fps, end/clip.fps)
clips.append(subclip)
final_clip = mp.concatenate_videoclips(clips)
```
7. 导出处理好的视频
```python
final_clip.write_videofile("output.mp4")
```
这只是一个基本的示例代码,实际应用中可能需要更复杂的算法和优化。
阅读全文
相关推荐
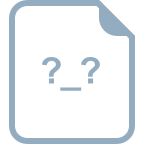
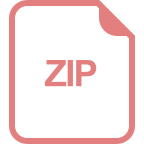
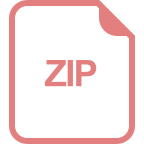











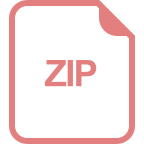
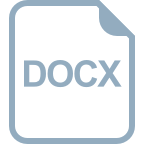
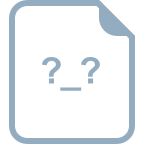
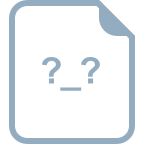
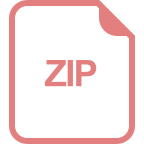