有一个接口使用multipartrequest.getfile方法获取文件,现在我想调用这个接口,我有一个File对象,请问如何把我File对象里对应的文件传输给该接口?
时间: 2024-01-24 08:20:46 浏览: 122
你可以使用`requests`库来发送请求,并使用`open`方法读取文件内容并将其作为文件对象传递给请求。示例代码如下:
```python
import requests
url = 'http://example.com/upload_file'
file_path = '/path/to/your/file.jpg'
with open(file_path, 'rb') as f:
files = {'file': f}
response = requests.post(url, files=files)
print(response.text)
```
在这个示例中,我们首先指定要上传到的URL和要上传的文件的路径。使用`open()`函数打开文件,将其作为二进制文件读取,并将其作为字典的值传递给`files`参数。
然后,我们使用`requests.post()`方法发送POST请求,并将文件作为`files`参数传递。最后,我们打印服务器的响应。
相关问题
public ResultBody saveMyCompanyInfo(MultipartRequest files, EcpBasCompany company) { List<MultipartFile> fileList = new ArrayList<>(); if(ObjectUtils.isNotEmpty(files)) { //获取文件 files.getMultiFileMap().forEach((key, multipartFiles) -> { MultipartFile multipartFile = multipartFiles.get(0); //传空的会有一个file的文件,先排除 if (!StringUtils.equals("file", key)) { fileList.add(multipartFile); } }); } return ecpBasCompanyService.saveMyCompany(fileList.toArray(new MultipartFile[fileList.size()]), company); }
这段代码是Java代码,它定义了一个名为saveMyCompanyInfo的函数,接受两个参数:MultipartRequest类型的文件和EcpBasCompany类型的公司。在函数内部,它首先创建了一个名为fileList的List变量,用于存储上传的文件。然后,它检查文件是否为空,如果不为空,遍历文件中的每个键值对,提取文件并添加到fileList中。最后,它调用了ecpBasCompanyService.saveMyCompany方法并将fileList和company参数作为参数传入。
MultipartFile对象如何创建
MultipartFile对象是Spring框架中用于处理文件上传的类,可以通过多种方式创建。其中最常见的方式是通过使用MultipartHttpServletRequest对象来获取MultipartFile对象。下面是一个示例代码:
```java
@PostMapping("/upload")
public String handleFileUpload(HttpServletRequest request) {
MultipartHttpServletRequest multipartRequest = (MultipartHttpServletRequest) request;
MultipartFile file = multipartRequest.getFile("file");
// 处理文件上传逻辑
return "File uploaded successfully!";
}
```
在上面的示例中,我们首先将HttpServletRequest对象强制转换成MultipartHttpServletRequest对象,然后通过调用getFile方法,传入文件对应的参数名获取MultipartFile对象。
还可以通过使用@RequestParam注解来直接声明MultipartFile参数,如下所示:
```java
@PostMapping("/upload")
public String handleFileUpload(@RequestParam("file") MultipartFile file) {
// 处理文件上传逻辑
return "File uploaded successfully!";
}
```
在上面的示例中,我们使用@RequestParam注解来声明文件参数,并将其类型设置为MultipartFile,Spring框架会自动将上传的文件转换成MultipartFile对象。
阅读全文
相关推荐



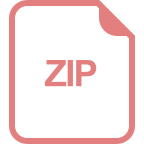









