该代码错误在哪?import java.util.ArrayList; import java.util.Arrays; import java.util.Objects; import java.util.Scanner; class PersonOverride{ private String name; private int age; private boolean gender; public PersonOverride(String name, int age, boolean gender) { this.name = name; this.age = age; this.gender = gender; } public PersonOverride() { this("default",1,true); } @Override public String toString() { return name + '-' + age + "-" + gender ; } @Override public boolean equals(Object o) { if (this == o) return true; if (o == null || getClass() != o.getClass()) return false; PersonOverride that = (PersonOverride) o; return age == that.age && gender == that.gender && Objects.equals(name, that.name); } @Override public int hashCode() { return Objects.hash(name, age, gender); } } public class Main { public static void main(String[] args) { Scanner sc = new Scanner(System.in); int n1=sc.nextInt(); PersonOverride[] persons1 = new PersonOverride[n1]; int n2 = sc.nextInt(); ArrayList<PersonOverride> p = new ArrayList<>(); for (int i = 0; i < n2; i++) { PersonOverride per = new PersonOverride(sc.next(),sc.nextInt(),sc.nextBoolean()); p.add(per); } for (int i = 0; i < p.size()-1; i++) { if(p.get(i).equals(p.get(i+1))){ p.remove(i); i--; } } PersonOverride[] persons2 = new PersonOverride[p.size()]; for (int i = 0; i < persons2.length; i++) { persons2[i]=p.get(i); } for (int i = 0; i < persons1.length; i++) { System.out.println(persons1[i].toString()); } for (int i = 0; i < persons2.length; i++) { System.out.println(persons2[i].toString()); } System.out.println(persons2.length); System.out.println(Arrays.toString(PersonOverride.class.getConstructors())); } }
时间: 2024-03-25 10:40:43 浏览: 92
该代码错误在于最后一段输出 `persons1` 数组时,该数组并没有进行初始化,因此会出现 `NullPointerException` 异常。具体来说,你在代码中使用了如下语句:
```java
for (int i = 0; i < persons1.length; i++) {
System.out.println(persons1[i].toString());
}
```
这段代码中 `persons1` 数组没有被初始化,因此其中的元素都是 `null`。在执行 `persons1[i].toString()` 时,由于 `persons1[i]` 为 `null`,因此会抛出 `NullPointerException` 异常。
解决该问题的方法有两种:一种是将 `persons1` 数组初始化,例如:
```java
PersonOverride[] persons1 = new PersonOverride[n1];
for (int i = 0; i < n1; i++) {
persons1[i] = new PersonOverride();
}
```
另一种是直接删掉这段输出 `persons1` 数组的代码。
相关问题
声明一个 Student 类,属性包括姓名、学号、总成绩;生成 10 个 Student 类对象,并放在一个一维数组中,编写方法按总成绩进行排序,将排序后的对象 分别保持在Vector 、ArrayList、 HashTable类型的对象中,并遍历显示其中 元素的信息。 程序设计参考框架如下: import java,util.Vector; import java.util.ArrayList; import java.util.Hashtable; import java.util.Enumeration; import java.util.Iterator; public class Ex71 { public static void main (String[] args){
public class Student implements Comparable<Student>{
private String name;
private int id;
private int totalScore;
public Student(String name, int id, int totalScore){
this.name = name;
this.id = id;
this.totalScore = totalScore;
}
public String getName(){
return name;
}
public int getId(){
return id;
}
public int getTotalScore(){
return totalScore;
}
public void setName(String name){
this.name = name;
}
public void setId(int id){
this.id = id;
}
public void setTotalScore(int totalScore){
this.totalScore = totalScore;
}
public int compareTo(Student s){
return this.totalScore - s.totalScore;
}
}
import java.util.Vector;
import java.util.ArrayList;
import java.util.Hashtable;
import java.util.Enumeration;
import java.util.Iterator;
public class Ex71 {
public static void main (String[] args){
Student[] students = new Student[10];
students[0] = new Student("Tom", 1001, 80);
students[1] = new Student("Jerry", 1002, 90);
students[2] = new Student("Alice", 1003, 85);
students[3] = new Student("Bob", 1004, 70);
students[4] = new Student("Peter", 1005, 95);
students[5] = new Student("Mary", 1006, 65);
students[6] = new Student("John", 1007, 75);
students[7] = new Student("Lucy", 1008, 88);
students[8] = new Student("David", 1009, 92);
students[9] = new Student("Lily", 1010, 78);
// sort by total score
Arrays.sort(students);
// put sorted objects into Vector
Vector<Student> vector = new Vector<Student>();
for(Student s : students){
vector.add(s);
}
// put sorted objects into ArrayList
ArrayList<Student> arrayList = new ArrayList<Student>();
for(Student s : students){
arrayList.add(s);
}
// put sorted objects into Hashtable
Hashtable<Integer, Student> hashtable = new Hashtable<Integer, Student>();
int i = 1;
for(Student s : students){
hashtable.put(i++, s);
}
// display elements in Vector
System.out.println("Elements in Vector:");
Enumeration<Student> enumeration = vector.elements();
while(enumeration.hasMoreElements()){
Student s = enumeration.nextElement();
System.out.println("Name: " + s.getName() + ", ID: " + s.getId() + ", Total Score: " + s.getTotalScore());
}
// display elements in ArrayList
System.out.println("Elements in ArrayList:");
Iterator<Student> iterator = arrayList.iterator();
while(iterator.hasNext()){
Student s = iterator.next();
System.out.println("Name: " + s.getName() + ", ID: " + s.getId() + ", Total Score: " + s.getTotalScore());
}
// display elements in Hashtable
System.out.println("Elements in Hashtable:");
Enumeration<Integer> keys = hashtable.keys();
while(keys.hasMoreElements()){
Integer key = keys.nextElement();
Student s = hashtable.get(key);
System.out.println("Name: " + s.getName() + ", ID: " + s.getId() + ", Total Score: " + s.getTotalScore());
}
}
}
import java.io.BufferedReader; import java.io.IOException; import java.io.InputStreamReader; import java.util.ArrayList; import java.util.Arrays; import java.util.Collection; import java.util.Collections; import java.util.LinkedList; import java.util.List; import java.util.Scanner; public class Main{ public static void main(String[] args) throws IOException { BufferedReader in=new BufferedReader(new InputStreamReader(System.in)); int n; double need; double sum=0; String s[]=in.readLine().split(" "); n=Integer.parseInt(s[0]); need=Double.parseDouble(s[1]); String amount[]=in.readLine().split(" "); String price[]=in.readLine().split(" "); ArrayList<Mooncake> cakes=new ArrayList<Mooncake>(); for(int i=0;i<n;i++){ Mooncake cake=new Mooncake(); cake.setAmount(Double.parseDouble(amount[i])); cake.setSales(Double.parseDouble(price[i])); cake.setValues(Double.parseDouble(price[i])/Double.parseDouble(amount[i])); cakes.add(cake); } Collections.sort(cakes); for(Mooncake c: cakes){ if(need>=c.amount){ need=need-c.amount; sum+=c.amount*c.values; } else{ sum+=need*c.values; need=0; } if(need==0){ break; } } System.out.printf("%.2f",sum); } static class Mooncake implements Comparable<Mooncake>{ double amount; double sales; double values; public double getAmount() { return amount; } public void setAmount(double amount) { this.amount = amount; } public double getSales() { return sales; } public void setSales(double sales) { this.sales = sales; } public double getValues() { return values; } public void setValues(double values) { this.values = values; } @Override public int compareTo(Mooncake arg0) { // TODO Auto-generated method stub return this.values>arg0.values?-1:1; } } }转c++
把Java代码转换成C++代码如下:
#include <iostream>
#include <algorithm>
#include <vector>
using namespace std;
struct Mooncake {
double amount;
double sales;
double values;
bool operator<(const Mooncake& c) const {
return this->values > c.values;
}
};
int main() {
int n;
double need, sum = 0;
cin >> n >> need;
vector<Mooncake> cakes(n);
for (int i = 0; i < n; i++) {
cin >> cakes[i].amount;
}
for (int i = 0; i < n; i++) {
double price;
cin >> price;
cakes[i].sales = price;
cakes[i].values = price / cakes[i].amount;
}
sort(cakes.begin(), cakes.end());
for (const auto& c : cakes) {
if (need >= c.amount) {
need = need - c.amount;
sum += c.amount * c.values;
}
else {
sum += need * c.values;
need = 0;
}
if (need == 0) {
break;
}
}
printf("%.2f", sum);
return 0;
}
阅读全文
相关推荐
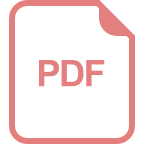
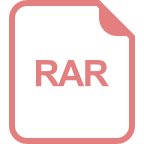
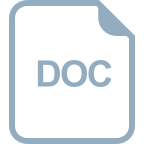
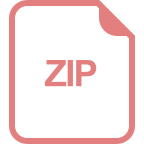
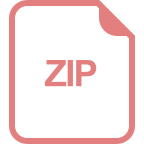
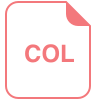











