编写一串C语言代码定义结构体Student,然后从终端上输入以下五个学生的学号,姓名,成绩并输出
时间: 2023-09-08 15:05:27 浏览: 188
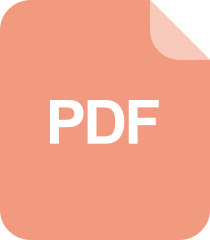
C语言利用结构体数组实现学生成绩管理系统

### 回答1:
int main() {
struct Student{
int id;
char name[20];
float score;
};
int i;
struct Student stu[5];
for(i=0;i<5;i++){
scanf("%d%s%f",&stu[i].id,stu[i].name,&stu[i].score);
}
for(i=0;i<5;i++){
printf("学号:%d\n姓名:%s\n成绩:%.2f\n",stu[i].id,stu[i].name,stu[i].score);
}
return 0;
}
### 回答2:
下面是一个用C语言编写的程序,定义了一个结构体Student,并从终端上输入五个学生的学号、姓名和成绩,并输出。
```c
#include <stdio.h>
// 定义结构体Student
struct Student {
int id;
char name[50];
float score;
};
int main() {
// 声明一个包含五个学生的数组
struct Student students[5];
// 从终端上输入每个学生的学号、姓名和成绩
for (int i = 0; i < 5; i++) {
printf("请输入第%d个学生的学号:", i + 1);
scanf("%d", &students[i].id);
printf("请输入第%d个学生的姓名:", i + 1);
scanf("%s", students[i].name);
printf("请输入第%d个学生的成绩:", i + 1);
scanf("%f", &students[i].score);
}
// 输出每个学生的学号、姓名和成绩
for (int i = 0; i < 5; i++) {
printf("第%d个学生的学号:%d\n", i + 1, students[i].id);
printf("第%d个学生的姓名:%s\n", i + 1, students[i].name);
printf("第%d个学生的成绩:%.2f\n\n", i + 1, students[i].score);
}
return 0;
}
```
以上代码中,我们首先定义了一个结构体Student,包含学号(id)、姓名(name)和成绩(score)三个成员变量。然后,在main函数中声明了一个包含五个学生的数组。接着,通过一个for循环,从终端上依次输入每个学生的学号、姓名和成绩,存储到数组中的相应位置。最后,再通过另一个for循环,依次输出每个学生的学号、姓名和成绩。
请注意,在输入学生的姓名时,我们使用%s来读取一个字符串,同时没有使用&操作符,因为name本身就是一个字符数组,而不是指针。另外,为了确保成绩的精度,我们使用了%.2f来控制输出的浮点数只保留两位小数。
希望以上代码能够满足你的需求。
### 回答3:
以下是一个用C语言编写的代码片段,实现了定义结构体Student,并从终端上输入五个学生的学号,姓名和成绩,并输出:
```c
#include <stdio.h>
#include <string.h>
// 定义结构体Student
struct Student {
int id;
char name[20];
float score;
};
int main() {
struct Student students[5]; // 定义一个存放五个学生对象的数组
// 从终端上输入五个学生的学号,姓名和成绩
for (int i = 0; i < 5; i++) {
printf("请输入第%d个学生的学号:", i + 1);
scanf("%d", &students[i].id);
fflush(stdin); // 清空输入缓冲区
printf("请输入第%d个学生的姓名:", i + 1);
fgets(students[i].name, sizeof(students[i].name), stdin);
students[i].name[strcspn(students[i].name, "\n")] = 0; // 去掉姓名中的换行符
printf("请输入第%d个学生的成绩:", i + 1);
scanf("%f", &students[i].score);
fflush(stdin); // 清空输入缓冲区
}
// 输出五个学生的学号,姓名和成绩
printf("\n五位学生的信息如下:\n");
for (int i = 0; i < 5; i++) {
printf("学号:%d\n", students[i].id);
printf("姓名:%s\n", students[i].name);
printf("成绩:%.2f\n\n", students[i].score);
}
return 0;
}
```
这段代码首先定义了一个结构体Student,其中包含学号(id)、姓名(name)和成绩(score)三个成员。接着在main函数中,定义了一个大小为5的Student类型的数组students,用于存放五个学生的信息。
然后通过for循环从终端上输入每个学生的学号、姓名和成绩,并保存到对应的students数组元素中。在输入姓名时,使用fgets和strcspn函数来处理输入带有换行符的字符串。
最后,通过另一个for循环,输出五个学生的学号、姓名和成绩。输出时使用printf函数来格式化输出。
编译并运行这段代码后,你可以在终端上依次输入五个学生的学号、姓名和成绩。然后程序会将这些信息存储在students数组中,并输出出来。
阅读全文
相关推荐
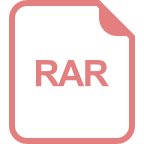
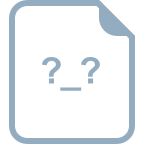















