t1 = time.time() # Note that compactness is defined differently because a grid is not used. Lower compactness for maskSLIC is equivalent segments = seg.slic(img, compactness=10, seed_type='nplace', mask=roi, n_segments=100, recompute_seeds=True, plot_examples=True, enforce_connectivity=True) print("Time: {:.2f} s".format(time.time() - t1)) for segment_id in np.unique(segments): mask = segments == segment_id masked_image = img.copy() masked_image[~mask] = 0 cv2.imwrite(r'I:\18Breakageratecalculation\mask-slic use\maskSLIC-master\result\result6\segment_{}.png'.format(segment_id), masked_image) plt.figure() plt.imshow(mark_boundaries(img, segments)) plt.contour(roi, contours=1, colors='red', linewidths=0.5) plt.axis('off') plt.savefig(r'I:\18Breakageratecalculation\mask-slic use\maskSLIC-master\result\result6\maskslic.png')提取每个子块图像的位置和大小信息
时间: 2024-03-22 12:38:16 浏览: 23
可以使用 `regionprops` 函数从分割结果中提取每个子块的位置和大小信息。具体步骤如下:
1. 将分割结果转换为标签图像,其中每个子块的像素值为其对应的标签值。
2. 使用 `regionprops` 函数提取标签图像中每个区域的属性信息,包括区域的位置、大小、像素值等。
3. 遍历所有区域,获取其位置和大小信息。
下面是示例代码:
```python
from skimage.measure import label, regionprops
# 将分割结果转换为标签图像
label_img = label(segments)
# 使用 regionprops 函数提取标签图像中每个区域的属性信息
props = regionprops(label_img)
# 遍历所有区域,获取其位置和大小信息
for prop in props:
# 获取区域的标签值、位置和大小信息
label_value = prop.label
bbox = prop.bbox # (min_row, min_col, max_row, max_col)
height = bbox[2] - bbox[0]
width = bbox[3] - bbox[1]
# 在原图上绘制该区域的边界框
cv2.rectangle(img, (bbox[1], bbox[0]), (bbox[3], bbox[2]), (0, 255, 0), 2)
# 输出该区域的位置和大小信息
print("Label: {}, Position: ({}, {}), Size: {} x {}".format(label_value, bbox[1], bbox[0], width, height))
```
其中,`bbox` 表示区域的最小外接矩形,其格式为 `(min_row, min_col, max_row, max_col)`,分别表示矩形左上角和右下角的行列坐标。`height` 和 `width` 分别表示矩形的高度和宽度。在实际使用中,可以根据需求选择输出或保存这些信息。
相关推荐
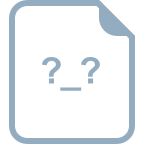
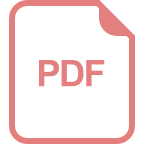
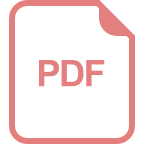








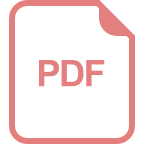
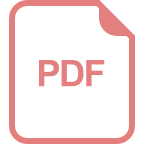
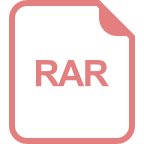
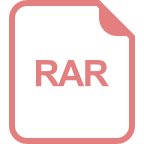
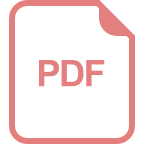