自己做过的球,如何运动的,如何和环境打交道,如何和用户的鼠标打交道。 写代码片断加以说明。
时间: 2024-02-01 15:14:56 浏览: 37
假设我开发了一个简单的足球游戏,下面是一些代码片段来说明球如何运动、与环境和用户鼠标进行交互:
1. 球如何运动:
```
// 定义足球对象
class Football {
constructor(x, y, radius) {
this.x = x;
this.y = y;
this.radius = radius;
this.speedX = 0;
this.speedY = 0;
}
// 更新球的位置
update() {
this.x += this.speedX;
this.y += this.speedY;
this.checkBoundaryCollision();
}
// 检测球是否与边界碰撞
checkBoundaryCollision() {
if (this.x + this.radius > canvas.width || this.x - this.radius < 0) {
this.speedX = -this.speedX;
}
if (this.y + this.radius > canvas.height || this.y - this.radius < 0) {
this.speedY = -this.speedY;
}
}
// 球被踢的时候计算速度
kick(angle, power) {
this.speedX = Math.cos(angle) * power;
this.speedY = Math.sin(angle) * power;
}
}
```
2. 球如何与环境打交道:
```
// 在游戏循环中更新球的位置
function gameLoop() {
ctx.clearRect(0, 0, canvas.width, canvas.height);
football.update();
football.draw();
window.requestAnimationFrame(gameLoop);
}
// 初始化足球对象并开始游戏循环
const football = new Football(50, 50, 10);
gameLoop();
```
3. 球如何与用户鼠标打交道:
```
// 在用户点击画布的时候将球踢出去
canvas.addEventListener('click', function(event) {
const angle = Math.atan2(event.clientY - football.y, event.clientX - football.x);
football.kick(angle, 10);
});
```
相关推荐
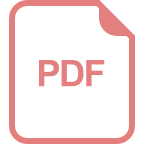
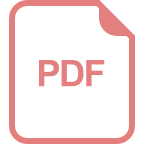














