stm32 usart2
时间: 2023-07-20 14:03:16 浏览: 42
STM32 USART2是一种通用异步收发传输器,常用于串口通信。它是STM32微控制器中的一个UART外设,可实现串口通信功能。
在使用USART2之前,需要先进行以下配置:
1.使能USART2外设时钟
2.配置USART2的GPIO引脚
3.配置USART2的波特率、数据位、停止位、校验位等参数
4.使能USART2外设
下面是一个使用USART2进行串口通信的示例代码:
```
#include "stm32f4xx.h"
void USART2_Init(void)
{
GPIO_InitTypeDef GPIO_InitStruct;
USART_InitTypeDef USART_InitStruct;
RCC_APB1PeriphClockCmd(RCC_APB1Periph_USART2, ENABLE);
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOA, ENABLE);
GPIO_InitStruct.GPIO_Pin = GPIO_Pin_2 | GPIO_Pin_3;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_AF;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStruct.GPIO_OType = GPIO_OType_PP;
GPIO_InitStruct.GPIO_PuPd = GPIO_PuPd_UP;
GPIO_Init(GPIOA, &GPIO_InitStruct);
GPIO_PinAFConfig(GPIOA, GPIO_PinSource2, GPIO_AF_USART2);
GPIO_PinAFConfig(GPIOA, GPIO_PinSource3, GPIO_AF_USART2);
USART_InitStruct.USART_BaudRate = 9600;
USART_InitStruct.USART_WordLength = USART_WordLength_8b;
USART_InitStruct.USART_StopBits = USART_StopBits_1;
USART_InitStruct.USART_Parity = USART_Parity_No;
USART_InitStruct.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStruct.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART2, &USART_InitStruct);
USART_Cmd(USART2, ENABLE);
}
void USART2_SendChar(char ch)
{
while(USART_GetFlagStatus(USART2, USART_FLAG_TXE) == RESET);
USART_SendData(USART2, (uint16_t)ch);
}
char USART2_ReceiveChar(void)
{
while(USART_GetFlagStatus(USART2, USART_FLAG_RXNE) == RESET);
return (char)USART_ReceiveData(USART2);
}
int main(void)
{
USART2_Init();
while(1)
{
char ch = USART2_ReceiveChar();
USART2_SendChar(ch);
}
}
```
上述代码中,我们首先进行了USART2的初始化,并配置了GPIO引脚为复用功能。然后设置了波特率为9600,数据位为8位,停止位为1位,无校验位,并使能了USART2外设。在main函数中,我们通过USART2_ReceiveChar函数读取串口接收到的数据,并通过USART2_SendChar函数发送回去。
相关推荐
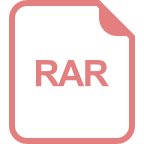














