用python创建一个序列窗体 窗体中有一个button按钮 单击该按钮后 就会弹出一个新窗体编程实现磁盘调度算法,并给出每种算法的平均寻道时间。
时间: 2024-02-24 20:58:35 浏览: 67
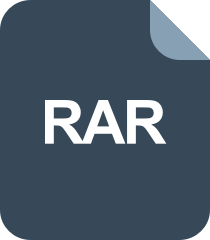
磁盘调度算法的实现
好的,以下是对应的 Python 代码:
```python
import tkinter as tk
from tkinter import messagebox
class NewWindow:
def __init__(self, algorithm):
self.algorithm = algorithm
self.window = tk.Toplevel()
self.window.title(algorithm)
self.window.geometry("300x150")
self.label = tk.Label(self.window, text="请输入磁盘访问序列:")
self.label.pack(pady=10)
self.entry = tk.Entry(self.window)
self.entry.pack()
self.button = tk.Button(self.window, text="开始计算", command=self.calculate)
self.button.pack(pady=10)
def calculate(self):
sequence = self.entry.get()
if sequence == "":
tk.messagebox.showwarning("提示", "请输入磁盘访问序列!")
return
sequence = list(map(int, sequence.split()))
if self.algorithm == "先来先服务算法":
result = fcfs(sequence)
elif self.algorithm == "最短寻道时间优先算法":
result = sstf(sequence)
elif self.algorithm == "电梯调度算法":
result = elevator(sequence)
else:
tk.messagebox.showwarning("提示", "未知的磁盘调度算法!")
return
tk.messagebox.showinfo("计算结果", "平均寻道时间:{:.2f}".format(result))
def fcfs(sequence):
n = len(sequence)
total = 0
for i in range(1, n):
total += abs(sequence[i] - sequence[i-1])
return total / n
def sstf(sequence):
n = len(sequence)
total = 0
curr_pos = sequence[0]
while sequence:
next_pos = min(sequence, key=lambda x: abs(x - curr_pos))
total += abs(next_pos - curr_pos)
curr_pos = next_pos
sequence.remove(next_pos)
return total / n
def elevator(sequence):
n = len(sequence)
total = 0
curr_pos = sequence[0]
direction = 1
while sequence:
if direction == 1:
next_pos = min([x for x in sequence if x >= curr_pos] + [max(sequence)])
else:
next_pos = max([x for x in sequence if x <= curr_pos] + [min(sequence)])
total += abs(next_pos - curr_pos)
curr_pos = next_pos
sequence.remove(next_pos)
if curr_pos == max(sequence):
direction = -1
elif curr_pos == min(sequence):
direction = 1
return total / n
class MainWindow:
def __init__(self):
self.root = tk.Tk()
self.root.title("磁盘调度算法")
self.root.geometry("300x150")
self.label = tk.Label(self.root, text="请选择磁盘调度算法:")
self.label.pack(pady=10)
self.fcfs_button = tk.Button(self.root, text="先来先服务算法", command=lambda: self.create_new_window("先来先服务算法"))
self.fcfs_button.pack(pady=5)
self.sstf_button = tk.Button(self.root, text="最短寻道时间优先算法", command=lambda: self.create_new_window("最短寻道时间优先算法"))
self.sstf_button.pack(pady=5)
self.elevator_button = tk.Button(self.root, text="电梯调度算法", command=lambda: self.create_new_window("电梯调度算法"))
self.elevator_button.pack(pady=5)
self.root.mainloop()
def create_new_window(self, algorithm):
NewWindow(algorithm)
if __name__ == "__main__":
MainWindow()
```
解释:
- `NewWindow` 类:用于创建新窗体,其中 `algorithm` 表示磁盘调度算法名称。在窗体中,用户可以输入磁盘访问序列,并通过点击按钮计算平均寻道时间。
- `calculate` 方法:用于计算平均寻道时间,并弹出提示框显示结果。具体的计算方式在 `fcfs`、`sstf` 和 `elevator` 函数中实现。
- `fcfs` 函数:实现先来先服务算法。
- `sstf` 函数:实现最短寻道时间优先算法。
- `elevator` 函数:实现电梯调度算法。
- `MainWindow` 类:主窗体,包含三个按钮,分别用于选择不同的磁盘调度算法。当用户点击按钮时,会创建一个新的 `NewWindow` 对象。
注意:在代码中,我们使用了 tkinter 库来创建窗体和按钮,并使用 messagebox 子模块来弹出提示框。如果您的电脑上还没有安装该库,可以使用以下命令进行安装:
```
pip install tkinter
```
阅读全文
相关推荐
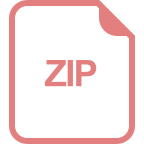
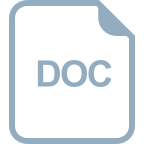




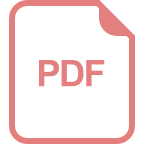
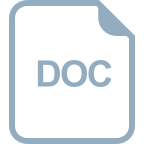









