QPushButton设置文字在图片下面
时间: 2024-03-05 19:47:38 浏览: 161
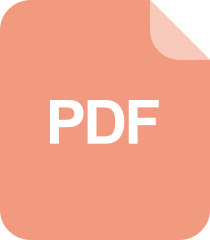
iOS - UIButton(UIEdgeInsets)/设置button上的文字和图片上下垂直居中对齐
您可以使用QPushButton的`setStyleSheet()`函数来设置样式表,以实现文字在图片下面的效果。以下是一个示例代码:
``` c++
QPushButton *button = new QPushButton();
button->setText("Button Text");
button->setIcon(QIcon("path/to/image.png"));
button->setIconSize(QSize(50, 50));
button->setStyleSheet("QPushButton {"
"border: none;"
"text-align: center;"
"}"
"QPushButton::icon {"
"margin-bottom: 10px;"
"}");
```
在上述代码中,`setStyleSheet()`函数设置了两个样式表规则。第一个规则设置了按钮的样式,将其边框设置为无,将文本居中对齐。第二个规则设置了按钮图标的样式,将其下边缘与文本之间的间距设置为10像素。
注意,上述代码中的`QIconSize()`函数用于设置图标的大小,以确保其与文本的间距正确。您需要根据自己的图标大小和文本长度进行调整。
阅读全文
相关推荐

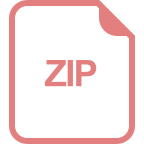
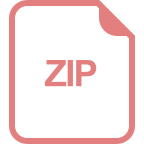
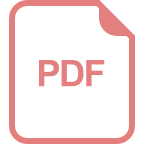
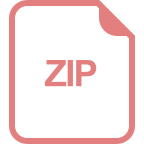
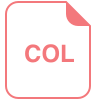
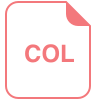
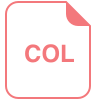
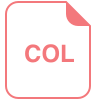





