如何使用&.post传递参数并用console.log显示
时间: 2023-06-12 08:03:52 浏览: 44
你可以通过以下步骤使用 ChitGPT:
1. 访问 CSDN 的 AI 对话页面:https://ai.csdn.net/baike/gpt
2. 在页面底部的输入框中输入你想要与 AI 对话的问题或话题。
3. 点击“发送”按钮,等待 AI 回复。
您也可以使用 Python 编程语言来集成 ChitGPT 到自己的应用程序中。CSDN 提供了 API 接口,您可以在自己的程序中调用该接口来实现与 AI 的对话。具体的使用方法请参考 CSDN AI 对话页面提供的 API 文档。
相关问题
axios.post传递params参数
axios.post方法可以通过两种方式传递参数:params和data。
如果要使用params参数,可以将参数作为一个对象传递给params属性。这样,参数将会被转换为URL的查询字符串附加在请求的URL后面。例如:
```javascript
axios.post('/api/endpoint', {
params: {
key1: value1,
key2: value2
}
})
```
这将发送一个POST请求到`/api/endpoint?key1=value1&key2=value2`。
另一种方式是使用data属性来传递参数。将参数作为一个对象传递给data属性,参数将会作为请求的主体数据发送。例如:
```javascript
axios.post('/api/endpoint', {
key1: value1,
key2: value2
})
```
这将发送一个POST请求到`/api/endpoint`,并且参数将会作为请求的主体数据发送。
需要注意的是,params参数适用于GET请求或者将参数附加在URL后面的场景,而data参数适用于POST请求或者将参数作为请求主体数据发送的场景。
requests.post传递的参数
requests.post() 方法用于发送 POST 请求,并接收服务器返回的响应。它可以传递以下参数:
1. url (必需): 要发送请求的 URL。
2. data: 要作为请求主体发送的数据,可以是字典、字符串或字节流。
3. json: 要作为请求主体发送的 JSON 数据。
4. headers: 要发送的请求标头,可以是字典类型。
5. params: 要在 URL 中添加的查询参数,可以是字典或字符串。
6. auth: (用户名, 密码) 元组,用于基本身份验证。
7. cookies: 要发送的 cookies,可以是 CookieJar 类型或字典类型。
8. timeout: 请求超时时间,以秒为单位。
9. files: 要上传的文件,可以是字典类型,包含文件名和文件对象。
10. proxies: 要使用的代理,可以是字典类型。
11. verify: 是否验证服务器的 SSL 证书。
12. allow_redirects: 是否允许重定向,默认为 True。
13. stream: 是否以流的方式获取响应内容,默认为 False。
这些是常用的参数,根据具体需求可以选择传递相应的参数。
相关推荐
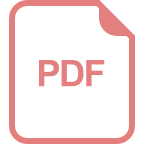














