根据点云最大最小边界叶脉线长度提取算法的代码python版本
时间: 2024-02-03 07:03:41 浏览: 21
以下是基于最大最小边界叶脉线长度的点云叶脉线提取算法的 Python 代码实现:
```python
import numpy as np
from scipy.spatial.distance import pdist, squareform
def find_nearest_neighbors(points, k=10):
"""
找到每个点的k个最近邻点
:param points: 点云数据,Nx3的numpy数组
:param k: 最近邻点数目
:return: 每个点的k个最近邻点的索引,Nxk的numpy数组
"""
dists = squareform(pdist(points))
nn_idx = np.argsort(dists)[:, 1:k+1]
return nn_idx
def compute_local_depth(points, nn_idx):
"""
计算每个点的局部深度
:param points: 点云数据,Nx3的numpy数组
:param nn_idx: 每个点的k个最近邻点的索引,Nxk的numpy数组
:return: 每个点的局部深度,Nx1的numpy数组
"""
dists = np.sqrt(np.sum((points[nn_idx] - np.expand_dims(points, axis=1))**2, axis=2))
depth = np.max(dists, axis=1)
return depth
def compute_boundary_length(points, nn_idx):
"""
计算每个点的最大最小边界叶脉线长度
:param points: 点云数据,Nx3的numpy数组
:param nn_idx: 每个点的k个最近邻点的索引,Nxk的numpy数组
:return: 每个点的最大最小边界叶脉线长度,Nx2的numpy数组
"""
dists = squareform(pdist(points))
nn_dists = np.sort(dists)[:, 1:3]
min_boundary_length = np.min(nn_dists, axis=1)
max_boundary_length = np.max(nn_dists, axis=1)
return np.vstack((min_boundary_length, max_boundary_length)).T
def extract_veins(points, k=10, depth_thresh=0.01, length_thresh=0.05):
"""
提取点云中的叶脉线
:param points: 点云数据,Nx3的numpy数组
:param k: 最近邻点数目
:param depth_thresh: 局部深度阈值
:param length_thresh: 最大最小边界叶脉线长度比例阈值
:return: 叶脉线点的索引
"""
nn_idx = find_nearest_neighbors(points, k)
depth = compute_local_depth(points, nn_idx)
boundary_length = compute_boundary_length(points, nn_idx)
is_vein = np.logical_and(depth < depth_thresh, boundary_length[:, 0] / boundary_length[:, 1] > length_thresh)
vein_idx = np.where(is_vein)[0]
return vein_idx
```
这里的 `points` 是一个 $N \times 3$ 的 numpy 数组,表示点云中的所有点;`k` 表示每个点的最近邻点数目,默认为 10;`depth_thresh` 表示局部深度的阈值,默认为 0.01;`length_thresh` 表示最大最小边界叶脉线长度比例的阈值,默认为 0.05。
函数 `find_nearest_neighbors` 用于找到每个点的最近邻点的索引;函数 `compute_local_depth` 计算每个点的局部深度;函数 `compute_boundary_length` 计算每个点的最大最小边界叶脉线长度;函数 `extract_veins` 则是整个算法的核心,提取出点云中的叶脉线点的索引。
相关推荐
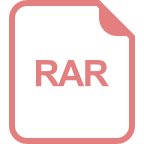
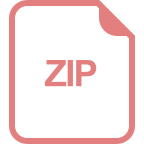














