RGB565转jpeg
时间: 2023-08-30 09:11:16 浏览: 226
RGB565是一种颜色编码格式,它使用16位来表示一个像素的颜色信息,其中5位用来表示红色分量,6位用来表示绿色分量,5位用来表示蓝色分量。而JPEG是一种图像压缩格式,它可以将图像数据压缩成较小的文件尺寸,同时保持图像质量。因此,将RGB565格式的图像转换成JPEG格式,需要进行以下步骤:
1. 将RGB565格式的图像数据解码成RGB24格式,即每个像素使用24位来表示颜色信息。这可以通过将每个像素的5位红色分量、6位绿色分量和5位蓝色分量进行扩展得到。
2. 将RGB24格式的图像数据压缩成JPEG格式。这可以使用JPEG编码库,如libjpeg等。
下面是一个使用libjpeg库将RGB565格式的图像转换成JPEG格式的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <jpeglib.h>
void rgb565_to_rgb24(unsigned char *rgb565_data, unsigned char *rgb24_data, int width, int height) {
int i, j;
unsigned short rgb565;
unsigned char r, g, b;
for (i = 0; i < height; i++) {
for (j = 0; j < width; j++) {
// get RGB565 color from data
rgb565 = (rgb565_data[(i * width + j) * 2] << 8) | rgb565_data[(i * width + j) * 2 + 1];
// expand to RGB24 format
r = (rgb565 >> 11) << 3;
g = ((rgb565 >> 5) & 0x3f) << 2;
b = (rgb565 & 0x1f) << 3;
rgb24_data[(i * width + j) * 3] = r;
rgb24_data[(i * width + j) * 3 + 1] = g;
rgb24_data[(i * width + j) * 3 + 2] = b;
}
}
}
int rgb24_to_jpeg(unsigned char *rgb24_data, int width, int height, int quality, char *filename) {
int i, j;
struct jpeg_compress_struct cinfo;
struct jpeg_error_mgr jerr;
JSAMPROW row_pointer[1];
FILE *outfile;
unsigned char *image_buffer;
int image_size;
// allocate memory for image buffer
image_size = width * height * 3;
image_buffer = (unsigned char *)malloc(image_size);
// initialize JPEG compression object
cinfo.err = jpeg_std_error(&jerr);
jpeg_create_compress(&cinfo);
// open output file
if ((outfile = fopen(filename, "wb")) == NULL) {
fprintf(stderr, "Can't open %s\n", filename);
return 0;
}
jpeg_stdio_dest(&cinfo, outfile);
// set image parameters
cinfo.image_width = width;
cinfo.image_height = height;
cinfo.input_components = 3;
cinfo.in_color_space = JCS_RGB;
jpeg_set_defaults(&cinfo);
jpeg_set_quality(&cinfo, quality, TRUE);
// start compression
jpeg_start_compress(&cinfo, TRUE);
// write image data
for (i = 0; i < height; i++) {
row_pointer[0] = &rgb24_data[i * width * 3];
jpeg_write_scanlines(&cinfo, row_pointer, 1);
}
// finish compression
jpeg_finish_compress(&cinfo);
// release resources
fclose(outfile);
jpeg_destroy_compress(&cinfo);
free(image_buffer);
return 1;
}
int main() {
int width = 320;
int height = 240;
int quality = 80;
unsigned char *rgb565_data = (unsigned char *)malloc(width * height * 2);
unsigned char *rgb24_data = (unsigned char *)malloc(width * height * 3);
char *filename = "output.jpg";
// TODO: fill rgb565_data with actual data
// convert RGB565 to RGB24
rgb565_to_rgb24(rgb565_data, rgb24_data, width, height);
// compress RGB24 to JPEG
rgb24_to_jpeg(rgb24_data, width, height, quality, filename);
// release resources
free(rgb565_data);
free(rgb24_data);
return 0;
}
```
以上代码仅供参考,实际应用中可能需要根据具体需求进行修改。
相关推荐
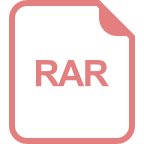














