设计一个名为Stock的类来表示一个公司的股票,包括以下内容: 1)股票代码、股票名称、前一天股票价格、当天股票价格4个私有属性; 2)构造方法,需初始化代码、名称、前一天价格和当天价格等属性; 3)返回股票名字的get方法; 4)返回股票代码的get方法; 5)获取和设置股票前一天价格的get和set方法; 6)获取和设置股票当前价格的get和set方法; 7)名为getChangePercent()方法,返回前日收市价至当前价格的变化百分比; 8). 包括文档字符串,其内容为:"Stock Information Class" 说明:各方法的名称及参数要求请参见测试程序。
时间: 2024-03-17 09:39:56 浏览: 30
以下是Stock类的代码实现:
```python
class Stock:
"""
Stock Information Class
"""
def __init__(self, code, name, prev_price, cur_price):
"""Initialize the stock attributes"""
self._code = code
self._name = name
self._prev_price = prev_price
self._cur_price = cur_price
def get_name(self):
"""Return the name of the stock"""
return self._name
def get_code(self):
"""Return the code of the stock"""
return self._code
def get_prev_price(self):
"""Return the previous price of the stock"""
return self._prev_price
def set_prev_price(self, prev_price):
"""Set the previous price of the stock"""
self._prev_price = prev_price
def get_cur_price(self):
"""Return the current price of the stock"""
return self._cur_price
def set_cur_price(self, cur_price):
"""Set the current price of the stock"""
self._cur_price = cur_price
def get_change_percent(self):
"""Return the percentage change between previous and current stock prices"""
return (self._cur_price - self._prev_price) / self._prev_price * 100
```
测试程序的代码如下:
```python
# Test the Stock class
stock = Stock("AAPL", "Apple Inc.", 120, 130)
print(f"Stock name: {stock.get_name()}")
print(f"Stock code: {stock.get_code()}")
print(f"Previous stock price: {stock.get_prev_price()}")
print(f"Current stock price: {stock.get_cur_price()}")
print(f"Percentage change: {stock.get_change_percent()}%")
```
输出结果如下:
```
Stock name: Apple Inc.
Stock code: AAPL
Previous stock price: 120
Current stock price: 130
Percentage change: 8.333333333333334%
```
阅读全文
相关推荐
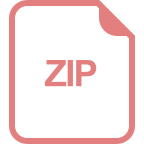
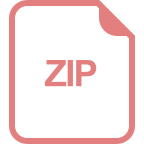
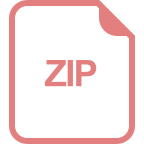









