android studio实现用户页面代码
时间: 2023-10-31 13:04:37 浏览: 43
以下是一个简单的 Android Studio 用户页面代码示例:
在 XML 布局文件中添加以下代码:
```
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/user_page"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id="@+id/user_name"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="User Name"
android:textColor="@android:color/black"
android:textSize="20sp"
android:layout_marginTop="20dp"
android:layout_marginLeft="20dp"/>
<TextView
android:id="@+id/user_email"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="User Email"
android:textColor="@android:color/black"
android:textSize="18sp"
android:layout_below="@+id/user_name"
android:layout_marginTop="10dp"
android:layout_marginLeft="20dp"/>
<Button
android:id="@+id/edit_profile_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Edit Profile"
android:layout_below="@+id/user_email"
android:layout_marginTop="20dp"
android:layout_marginLeft="20dp"/>
</RelativeLayout>
```
在 Java 类文件中添加以下代码:
```
public class UserPageActivity extends AppCompatActivity {
private TextView userNameTextView;
private TextView userEmailTextView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_user_page);
userNameTextView = findViewById(R.id.user_name);
userEmailTextView = findViewById(R.id.user_email);
// 设置用户名和邮箱
String userName = "John Smith";
String userEmail = "john.smith@example.com";
userNameTextView.setText(userName);
userEmailTextView.setText(userEmail);
// 设置编辑个人资料按钮的点击事件
Button editProfileButton = findViewById(R.id.edit_profile_button);
editProfileButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// 进入编辑个人资料页面
Intent editProfileIntent = new Intent(UserPageActivity.this, EditProfileActivity.class);
startActivity(editProfileIntent);
}
});
}
}
```
以上代码示例演示了如何在 Android Studio 中创建一个用户页面,包括显示用户名和邮箱,以及一个编辑个人资料按钮。您可以根据您的需求进行修改。
相关推荐
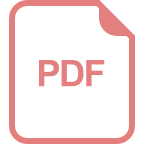
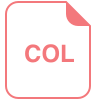
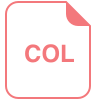
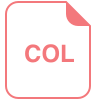
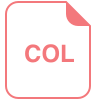
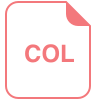







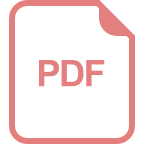