#include <iostream> #include <string.h> using namespace std; #define STR_LEN 80 //读入一行存入字符数组str,长度不超过maxLength void readline(char str[], int maxLength); //判断字符串str是否是回文 bool isPalindrome(char str[]); int main() { char str[STR_LEN + 1]; // cout << "Please input a string with length <= " << STR_LEN << ":"; readline(str, STR_LEN); if (isPalindrome(str)) { cout << "Yes" << endl; } else { cout << "No" << endl; } return 0; } //读入一行存入字符数组str,长度不超过maxLength void readline(char str[], int maxLength) { int i = 0; while (i < maxLength) { char ch = cin.get(); if (ch != '\n' && ch != '\r') { str[i] = ch; } else { break; } i++; } str[i] = '\0'; } //判断字符串str是否是回文 bool isPalindrome(char str[]) { int len = strlen(str); int left = 0, right = len - 1; while (left < right) { // 忽略英文字符的大小写 if (isalpha(str[left]) && isalpha(str[right])) { if (tolower(str[left]) != tolower(str[right])) { return false; } left++; right--; } else if (!isalpha(str[left])) { left++; } else if (!isalpha(str[right])) { right--; } } return true; }这个程序输入“国防科技大学”时,输出为"Yes",可是他本应该输出“No”,怎样修改这个程序使其可以正确判断输入的中文是否是回文
时间: 2024-01-05 09:02:17 浏览: 73
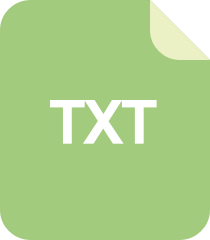
使用include <iostream>时一定要加using namespace std
这个程序只考虑了英文字符的大小写,而没有考虑中文字符。中文字符需要特殊处理,因为一个中文字符占用两个字节。可以使用宽字符类型wchar_t来存储中文字符。
下面是修改后的程序:
```cpp
#include <iostream>
#include <string.h>
#include <locale.h>
using namespace std;
#define STR_LEN 80
//读入一行存入字符数组str,长度不超过maxLength
void readline(char str[], int maxLength);
//判断字符串str是否是回文
bool isPalindrome(char str[]);
int main()
{
char str[STR_LEN + 1];
// 设置本地化环境,以便支持中文字符
setlocale(LC_ALL, "");
cout << "请输入一个长度不超过" << STR_LEN << "的字符串:";
readline(str, STR_LEN);
if (isPalindrome(str))
{
cout << "是回文" << endl;
}
else
{
cout << "不是回文" << endl;
}
return 0;
}
//读入一行存入字符数组str,长度不超过maxLength
void readline(char str[], int maxLength)
{
int i = 0;
while (i < maxLength)
{
char ch = cin.get();
if (ch != '\n' && ch != '\r')
{
str[i] = ch;
}
else
{
break;
}
i++;
}
str[i] = '\0';
}
//判断字符串str是否是回文
bool isPalindrome(char str[])
{
int len = strlen(str);
int left = 0, right = len - 1;
while (left < right)
{
if (iswalpha(str[left]) && iswalpha(str[right]))
{
if (towlower(str[left]) != towlower(str[right]))
{
return false;
}
left++;
right--;
}
else if (!iswalpha(str[left]))
{
left++;
}
else if (!iswalpha(str[right]))
{
right--;
}
else
{
// 如果是中文字符,需要判断两个字节是否相等
if (str[left] != str[right])
{
return false;
}
left += 2;
right -= 2;
}
}
return true;
}
```
修改的地方包括:
1. 在程序开头添加了头文件locale.h,以便设置本地化环境。
2. 在主函数中添加了setlocale函数,设置本地化环境为当前系统的默认环境。
3. 在读取字符串时,使用cin.get函数读取字符,而不是getline函数。
4. 在判断字符是否是字母时,使用iswalpha函数代替isalpha函数。
5. 在处理中文字符时,需要判断两个字节是否相等,因此需要将左右指针分别加上2。
阅读全文
相关推荐
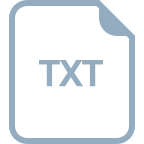





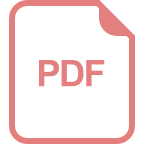
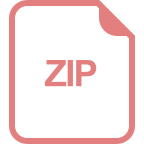
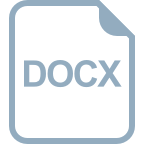
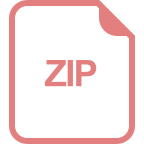
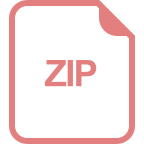
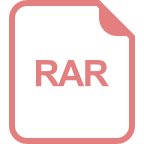