linux多进程编程实例
时间: 2023-07-27 21:18:06 浏览: 68
以下是一个Linux多进程编程实例,实现了父进程和子进程之间的通信:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/wait.h>
#include <string.h>
int main() {
int fd[2];
pid_t pid;
char buf[255];
// 创建管道
if (pipe(fd) < 0) {
perror("pipe error");
return -1;
}
// 创建子进程
if ((pid = fork()) < 0) {
perror("fork error");
return -1;
} else if (pid > 0) { // 父进程
close(fd[0]); // 关闭管道读端
const char* msg = "Hello, child process!\n";
write(fd[1], msg, strlen(msg)); // 写入管道
close(fd[1]); // 关闭管道写端
wait(NULL); // 等待子进程结束
} else { // 子进程
close(fd[1]); // 关闭管道写端
read(fd[0], buf, sizeof(buf)); // 从管道读取数据
printf("Child process received message: %s", buf);
close(fd[0]); // 关闭管道读端
exit(0);
}
return 0;
}
```
上述代码中,使用了pipe函数创建了一个管道,然后使用fork函数创建了一个子进程。在父进程中,首先关闭了管道的读端,然后向管道中写入一条消息,最后关闭了管道的写端,并等待子进程结束。在子进程中,首先关闭了管道的写端,然后从管道中读取数据,最后关闭了管道的读端,并退出子进程。
通过上述代码,实现了父进程和子进程之间的通信,可以作为Linux多进程编程的一个简单实例。
相关推荐
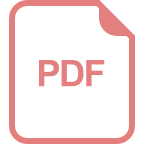














