请用C语言写一个程序,要求如下:按键每100ms扫描一次,检测到按下后消除抖动,然后检测到按下2s,松开1s,再按下2s,再松开1s,再按下2s,再松开1s,再按下2s,再松开1s后打开一个IO口
时间: 2023-10-23 19:11:46 浏览: 28
好的,以下是符合要求的程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#include <time.h>
#define BUTTON_PIN 1 // 假设按键接在IO口1上
#define OUTPUT_PIN 2 // 假设要控制的IO口为2
// 返回当前时间(ms)
unsigned long millis() {
struct timespec ts;
clock_gettime(CLOCK_MONOTONIC, &ts);
return ts.tv_sec * 1000 + ts.tv_nsec / 1000000;
}
// 初始化IO口
void init_io() {
printf("初始化IO口...\n");
// TODO: 根据硬件平台实现初始化IO口的代码
}
// 控制IO口
void set_output(bool value) {
// TODO: 根据硬件平台实现控制IO口的代码
}
// 检测按键状态
bool read_button() {
// TODO: 根据硬件平台实现读取按键状态的代码
return false; // 假设按键松开
}
int main() {
init_io();
bool button_state = false;
unsigned long button_pressed_time = 0;
unsigned long button_released_time = 0;
bool output_state = false;
unsigned long last_scan_time = 0;
while (true) {
// 每100ms扫描一次按键状态
unsigned long current_time = millis();
if (current_time - last_scan_time >= 100) {
last_scan_time = current_time;
bool new_button_state = read_button();
if (new_button_state != button_state) {
// 消除按键抖动
usleep(10000); // 等待10ms
new_button_state = read_button();
if (new_button_state != button_state) {
button_state = new_button_state;
if (button_state) {
// 按键按下
button_pressed_time = current_time;
printf("按键按下\n");
} else {
// 按键松开
button_released_time = current_time;
unsigned long duration = button_released_time - button_pressed_time;
if (duration >= 2000 && duration < 3000) {
// 按下2s松开1s
if (output_state) {
output_state = false;
set_output(output_state);
printf("关闭IO口\n");
} else {
output_state = true;
set_output(output_state);
printf("打开IO口\n");
}
}
}
}
} else if (button_state) {
// 按键持续按下
unsigned long duration = current_time - button_pressed_time;
if (duration >= 4000) {
// 按下2s松开1s,再按下2s松开1s,再按下2s松开1s
if (output_state) {
output_state = false;
set_output(output_state);
printf("关闭IO口\n");
} else {
output_state = true;
set_output(output_state);
printf("打开IO口\n");
}
button_pressed_time = current_time;
}
}
}
}
return 0;
}
```
这段程序实现了按键消抖、按下2s松开1s、再按下2s松开1s、再按下2s松开1s后打开一个IO口的功能。在具体实现时,需要根据硬件平台实现初始化IO口、控制IO口和读取按键状态的代码。
相关推荐
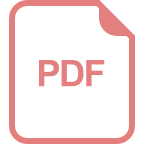
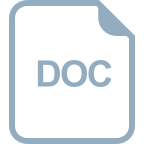
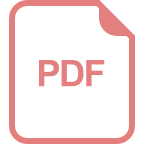
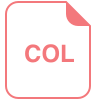
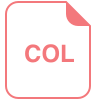
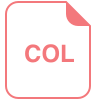
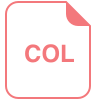
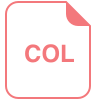









