统计一个文本文件中的字符(包括空格)数、单词数和行的数目。
时间: 2024-05-14 16:13:27 浏览: 78
可以使用Python编程实现这个功能。具体的实现方法如下:
```
# 定义函数,统计文本文件中的字符数、单词数和行数
def count_words(filename):
# 打开文件
with open(filename, 'r') as file:
# 统计字符数和单词数
num_chars = 0
num_words = 0
for line in file:
num_chars += len(line)
num_words += len(line.split())
# 统计行数
num_lines = len(file.readlines())
# 返回统计结果
return (num_chars, num_words, num_lines)
# 调用函数,统计指定文本文件中的字符数、单词数和行数
filename = 'test.txt'
result = count_words(filename)
print("字符数 = ", result[0])
print("单词数 = ", result[1])
print("行数 = ", result[2])
```
当然,实际上还需要考虑一些细节问题,比如文件不存在的情况、文件编码等等,但是代码有些冗长,为了演示的简洁性我就不一一列举了。
相关问题
编写程序,统计一个文本文件中的字符(包括空格)数、单词数和行的数目。
要编写一个程序来统计文本文件中的字符数、单词数和行数,你可以使用Java的IO流和正则表达式来完成这个任务。以下是一个简单的示例,该程序读取文本文件并逐行处理:
```java
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class FileCounter {
public static void main(String[] args) {
String filePath = "path_to_your_text_file.txt"; // 替换为你的文本文件路径
int characterCount = countCharacters(filePath);
int wordCount = countWords(filePath);
int lineCount = countLines(filePath);
System.out.println("Character Count: " + characterCount);
System.out.println("Word Count: " + wordCount);
System.out.println("Line Count: " + lineCount);
}
private static int countCharacters(String filePath) {
try (BufferedReader br = new BufferedReader(new FileReader(filePath))) {
int sum = 0;
String line;
while ((line = br.readLine()) != null) {
sum += line.length();
}
return sum;
} catch (IOException e) {
throw new RuntimeException("Error reading file", e);
}
}
private static int countWords(String filePath) {
try (BufferedReader br = new BufferedReader(new FileReader(filePath))) {
int wordCount = 0;
String line;
while ((line = br.readLine()) != null) {
wordCount += line.split("\\s+").length; // 使用正则表达式分割单词,忽略标点符号
}
return wordCount;
} catch (IOException e) {
throw new RuntimeException("Error reading file", e);
}
}
private static int countLines(String filePath) {
try (BufferedReader br = new BufferedReader(new FileReader(filePath))) {
int lineCount = 0;
String line;
while ((line = br.readLine()) != null) {
lineCount++;
}
return lineCount;
} catch (IOException e) {
throw new RuntimeException("Error reading file", e);
}
}
}
```
在这个例子中,我们定义了三个辅助方法:`countCharacters`计算字符数,`countWords`计算单词数(不包含标点),`countLines`计算行数。每个方法都使用`BufferedReader`逐行读取文件,然后累加计数。
请确保将`path_to_your_text_file.txt`替换为你实际要处理的文本文件路径。运行此程序后,它将在控制台上输出给定文本文件的字符数、单词数和行数。
用Java编写程序统计一个文本文件中的字符(包括空格)数、单词数和行的数目。
答案:可以使用Java中的File类和Scanner类来读取文本文件,统计字符数和行数,可以使用String类中的split()方法来分割单词并统计单词数。具体代码可以参考以下示例:
```java
import java.io.File;
import java.util.Scanner;
public class CharacterWordLineCounter {
public static void main(String[] args) {
try {
// 创建File对象
File file = new File("path/to/textfile.txt");
// 创建Scanner对象读取文件
Scanner scanner = new Scanner(file);
int characterCount = 0;
int wordCount = 0;
int lineCount = 0;
// 逐行读取文件内容
while (scanner.hasNextLine()) {
String line = scanner.nextLine();
// 统计行数
lineCount++;
// 统计字符数
characterCount += line.length();
// 使用正则表达式分割单词
String[] words = line.split("\\s+");
wordCount += words.length;
}
// 输出统计结果
System.out.println("字符数:" + characterCount);
System.out.println("单词数:" + wordCount);
System.out.println("行数:" + lineCount);
// 关闭文件输入流
scanner.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
当然,为了更加实用,也可以将程序写成一个方法,并且加上参数传递文件路径,即可快速实现在多个文本文件中的使用。
阅读全文
相关推荐
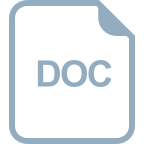
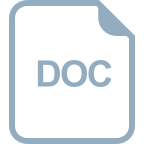
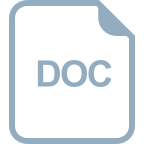












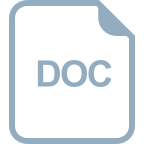
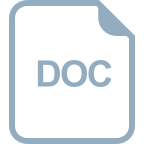