java接口返回json数据格式
时间: 2023-06-16 08:07:22 浏览: 128
要让 Java 接口返回 JSON 数据格式,你可以使用任何一个 JSON 库,比如 Jackson、Gson 或者 Fastjson。以下是使用 Jackson 库的示例代码:
首先,确保你的项目中已经引入了 Jackson 库的依赖。如果使用 Maven 管理项目依赖,可以在 pom.xml 文件中添加以下依赖:
```xml
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.12.3</version>
</dependency>
```
然后,在你的 Controller 中,可以按照如下方式编写代码:
```java
import com.fasterxml.jackson.databind.ObjectMapper;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class MyController {
@Autowired
private ObjectMapper objectMapper;
@GetMapping("/my-api")
public MyResponse myApi() {
MyResponse response = new MyResponse();
response.setCode(200);
response.setMessage("Success");
// 设置其它字段...
return response;
}
public static class MyResponse {
private int code;
private String message;
// 其它字段...
// 省略 getter 和 setter 方法...
public String toJson() {
try {
return objectMapper.writeValueAsString(this);
} catch (Exception e) {
throw new RuntimeException(e);
}
}
}
}
```
以上代码中,我们使用 Jackson 库的 ObjectMapper 对象将 Java 对象转换为 JSON 字符串。在 MyResponse 类中,我们添加了 toJson() 方法,该方法将 MyResponse 对象转换为 JSON 字符串。
当客户端请求 /my-api 接口时,MyController 的 myApi() 方法返回 MyResponse 对象,并且 MyResponse 对象会被自动转换为 JSON 格式的数据返回给客户端。如果你想手动序列化对象为 JSON 字符串,可以调用 MyResponse.toJson() 方法。
阅读全文
相关推荐
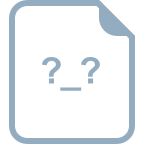

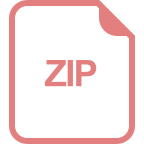
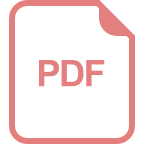
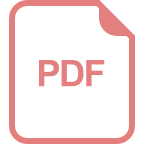
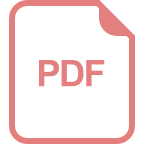
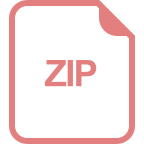
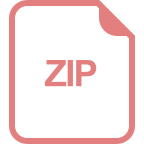
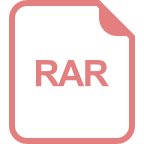
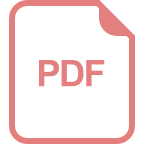
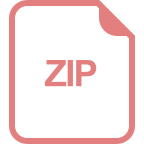
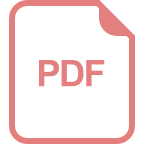
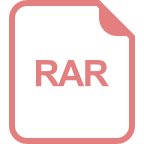
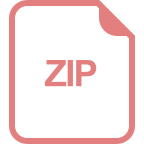
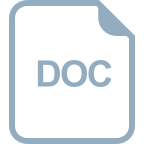
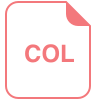
