基本信息管理模块的主要功能有学生信息的添加、删除、修改、显示和学生数据的导入导出,学生成绩管理模块的主要功能有统计课程最高分、最低分和平均分。要求按照面向对象思维进行编写,按照以下模式完成: 1、设计一个学生类,包含学号、姓名、语文成绩、数学成绩和英语成绩等数据成员。 2、引入OS模块,用于导入导出路径文件信息 3、设计一个学生管理类,用于定义各个模块的具体功能函数。 4、设计一个主菜单,两个子菜单,分别管理学生基本信息和学生成绩信息
时间: 2024-04-21 12:27:03 浏览: 55
好的,你的问题是如何设计一个学生成绩管理系统,对吧?基于面向对象的思想,你可以按照以下步骤进行编写:
1. 设计一个学生类,包含学号、姓名、语文成绩、数学成绩和英语成绩等数据成员。这个类可以定义在一个单独的文件中,例如student.py:
```python
class Student:
def __init__(self, id, name, chinese_score, math_score, english_score):
self.id = id
self.name = name
self.chinese_score = chinese_score
self.math_score = math_score
self.english_score = english_score
```
2. 引入OS模块,用于导入导出路径文件信息。在管理类中定义一个导入和导出的函数,例如:
```python
import os
class StudentManager:
def __init__(self):
self.students = []
def import_from_file(self, file_path):
if not os.path.exists(file_path):
print("File not exist.")
return
with open(file_path, "r") as f:
lines = f.readlines()
for line in lines:
data = line.strip().split(",")
student = Student(data[0], data[1], int(data[2]), int(data[3]), int(data[4]))
self.students.append(student)
def export_to_file(self, file_path):
with open(file_path, "w") as f:
for student in self.students:
f.write("{},{},{},{},{}\n".format(student.id, student.name, student.chinese_score, student.math_score, student.english_score))
```
3. 设计一个学生管理类,用于定义各个模块的具体功能函数。这个类可以包含添加、删除、修改、显示和统计成绩等函数。例如:
```python
class StudentManager:
def __init__(self):
self.students = []
def add_student(self, student):
self.students.append(student)
def remove_student(self, student_id):
for student in self.students:
if student.id == student_id:
self.students.remove(student)
return
def update_student(self, student_id, name, chinese_score, math_score, english_score):
for student in self.students:
if student.id == student_id:
student.name = name
student.chinese_score = chinese_score
student.math_score = math_score
student.english_score = english_score
return
def show_students(self):
for student in self.students:
print("ID:{} Name:{} Chinese:{} Math:{} English:{}".format(student.id, student.name, student.chinese_score, student.math_score, student.english_score))
def calculate_scores(self):
chinese_scores = []
math_scores = []
english_scores = []
for student in self.students:
chinese_scores.append(student.chinese_score)
math_scores.append(student.math_score)
english_scores.append(student.english_score)
max_chinese_score = max(chinese_scores)
min_chinese_score = min(chinese_scores)
average_chinese_score = sum(chinese_scores) / len(chinese_scores)
max_math_score = max(math_scores)
min_math_score = min(math_scores)
average_math_score = sum(math_scores) / len(math_scores)
max_english_score = max(english_scores)
min_english_score = min(english_scores)
average_english_score = sum(english_scores) / len(english_scores)
print("Chinese: Max:{} Min:{} Average:{}".format(max_chinese_score, min_chinese_score, average_chinese_score))
print("Math: Max:{} Min:{} Average:{}".format(max_math_score, min_math_score, average_math_score))
print("English: Max:{} Min:{} Average:{}".format(max_english_score, min_english_score, average_english_score))
```
4. 设计一个主菜单,两个子菜单,分别管理学生基本信息和学生成绩信息。例如:
```python
def main():
manager = StudentManager()
while True:
print("=== Main Menu ===")
print("1. Student Basic Information Management")
print("2. Student Score Management")
print("3. Quit")
choice = input("Please enter your choice:")
if choice == "1":
while True:
print("=== Student Basic Information Management ===")
print("1. Add Student")
print("2. Remove Student")
print("3. Update Student")
print("4. Show Students")
print("5. Import from File")
print("6. Export to File")
print("7. Back")
choice = input("Please enter your choice:")
if choice == "1":
id = input("Please enter student ID:")
name = input("Please enter student name:")
chinese_score = int(input("Please enter Chinese score:"))
math_score = int(input("Please enter Math score:"))
english_score = int(input("Please enter English score:"))
student = Student(id, name, chinese_score, math_score, english_score)
manager.add_student(student)
elif choice == "2":
student_id = input("Please enter the ID of the student you want to remove:")
manager.remove_student(student_id)
elif choice == "3":
student_id = input("Please enter the ID of the student you want to update:")
name = input("Please enter updated name:")
chinese_score = int(input("Please enter updated Chinese score:"))
math_score = int(input("Please enter updated Math score:"))
english_score = int(input("Please enter updated English score:"))
manager.update_student(student_id, name, chinese_score, math_score, english_score)
elif choice == "4":
manager.show_students()
elif choice == "5":
file_path = input("Please enter the file path:")
manager.import_from_file(file_path)
elif choice == "6":
file_path = input("Please enter the file path:")
manager.export_to_file(file_path)
elif choice == "7":
break
else:
print("Invalid choice.")
elif choice == "2":
while True:
print("=== Student Score Management ===")
print("1. Calculate Scores")
print("2. Back")
choice = input("Please enter your choice:")
if choice == "1":
manager.calculate_scores()
elif choice == "2":
break
else:
print("Invalid choice.")
elif choice == "3":
print("Quit.")
break
else:
print("Invalid choice.")
if __name__ == "__main__":
main()
```
这样,一个基于面向对象思想的学生成绩管理系统就完成了。
相关推荐
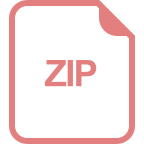















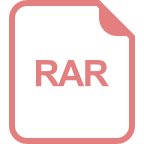
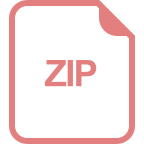