C++实现文件的AES和DES加密
时间: 2024-05-09 13:14:24 浏览: 7
实现文件的AES和DES加密需要以下步骤:
1. 读取文件数据
2. 对数据进行填充,使其满足加密算法的要求
3. 设置加密算法的参数,如密钥、加密模式、填充方式等
4. 执行加密操作
5. 将加密后的数据写入文件
下面是一个使用 OpenSSL 库实现文件的 AES 和 DES 加密的示例代码:
```c
#include <stdio.h>
#include <openssl/evp.h>
#define BUFFER_SIZE 1024
void encrypt_file_aes(const char* input_file, const char* output_file, const unsigned char* key, const unsigned char* iv) {
// 打开输入文件
FILE* in = fopen(input_file, "rb");
if (in == NULL) {
printf("无法打开输入文件 %s\n", input_file);
return;
}
// 打开输出文件
FILE* out = fopen(output_file, "wb");
if (out == NULL) {
fclose(in);
printf("无法打开输出文件 %s\n", output_file);
return;
}
// 创建 AES 加密上下文
EVP_CIPHER_CTX* ctx = EVP_CIPHER_CTX_new();
if (ctx == NULL) {
fclose(in);
fclose(out);
printf("无法创建加密上下文\n");
return;
}
// 初始化 AES 加密上下文
if (EVP_EncryptInit_ex(ctx, EVP_aes_256_cbc(), NULL, key, iv) != 1) {
fclose(in);
fclose(out);
EVP_CIPHER_CTX_free(ctx);
printf("无法初始化加密上下文\n");
return;
}
unsigned char inbuf[BUFFER_SIZE];
unsigned char outbuf[BUFFER_SIZE + EVP_MAX_BLOCK_LENGTH];
int inlen, outlen;
// 循环读取、加密和写入文件数据
do {
inlen = fread(inbuf, 1, BUFFER_SIZE, in);
if (EVP_EncryptUpdate(ctx, outbuf, &outlen, inbuf, inlen) != 1) {
fclose(in);
fclose(out);
EVP_CIPHER_CTX_free(ctx);
printf("无法加密数据\n");
return;
}
fwrite(outbuf, 1, outlen, out);
} while (inlen == BUFFER_SIZE);
// 结束加密操作
if (EVP_EncryptFinal_ex(ctx, outbuf, &outlen) != 1) {
fclose(in);
fclose(out);
EVP_CIPHER_CTX_free(ctx);
printf("无法结束加密操作\n");
return;
}
fwrite(outbuf, 1, outlen, out);
// 释放资源
fclose(in);
fclose(out);
EVP_CIPHER_CTX_free(ctx);
}
void decrypt_file_aes(const char* input_file, const char* output_file, const unsigned char* key, const unsigned char* iv) {
// 打开输入文件
FILE* in = fopen(input_file, "rb");
if (in == NULL) {
printf("无法打开输入文件 %s\n", input_file);
return;
}
// 打开输出文件
FILE* out = fopen(output_file, "wb");
if (out == NULL) {
fclose(in);
printf("无法打开输出文件 %s\n", output_file);
return;
}
// 创建 AES 解密上下文
EVP_CIPHER_CTX* ctx = EVP_CIPHER_CTX_new();
if (ctx == NULL) {
fclose(in);
fclose(out);
printf("无法创建解密上下文\n");
return;
}
// 初始化 AES 解密上下文
if (EVP_DecryptInit_ex(ctx, EVP_aes_256_cbc(), NULL, key, iv) != 1) {
fclose(in);
fclose(out);
EVP_CIPHER_CTX_free(ctx);
printf("无法初始化解密上下文\n");
return;
}
unsigned char inbuf[BUFFER_SIZE + EVP_MAX_BLOCK_LENGTH];
unsigned char outbuf[BUFFER_SIZE];
int inlen, outlen;
// 循环读取、解密和写入文件数据
do {
inlen = fread(inbuf, 1, BUFFER_SIZE + EVP_MAX_BLOCK_LENGTH, in);
if (EVP_DecryptUpdate(ctx, outbuf, &outlen, inbuf, inlen) != 1) {
fclose(in);
fclose(out);
EVP_CIPHER_CTX_free(ctx);
printf("无法解密数据\n");
return;
}
fwrite(outbuf, 1, outlen, out);
} while (inlen == BUFFER_SIZE + EVP_MAX_BLOCK_LENGTH);
// 结束解密操作
if (EVP_DecryptFinal_ex(ctx, outbuf, &outlen) != 1) {
fclose(in);
fclose(out);
EVP_CIPHER_CTX_free(ctx);
printf("无法结束解密操作\n");
return;
}
fwrite(outbuf, 1, outlen, out);
// 释放资源
fclose(in);
fclose(out);
EVP_CIPHER_CTX_free(ctx);
}
void encrypt_file_des(const char* input_file, const char* output_file, const unsigned char* key, const unsigned char* iv) {
// 打开输入文件
FILE* in = fopen(input_file, "rb");
if (in == NULL) {
printf("无法打开输入文件 %s\n", input_file);
return;
}
// 打开输出文件
FILE* out = fopen(output_file, "wb");
if (out == NULL) {
fclose(in);
printf("无法打开输出文件 %s\n", output_file);
return;
}
// 创建 DES 加密上下文
EVP_CIPHER_CTX* ctx = EVP_CIPHER_CTX_new();
if (ctx == NULL) {
fclose(in);
fclose(out);
printf("无法创建加密上下文\n");
return;
}
// 初始化 DES 加密上下文
if (EVP_EncryptInit_ex(ctx, EVP_des_cbc(), NULL, key, iv) != 1) {
fclose(in);
fclose(out);
EVP_CIPHER_CTX_free(ctx);
printf("无法初始化加密上下文\n");
return;
}
unsigned char inbuf[BUFFER_SIZE];
unsigned char outbuf[BUFFER_SIZE + EVP_MAX_BLOCK_LENGTH];
int inlen, outlen;
// 循环读取、加密和写入文件数据
do {
inlen = fread(inbuf, 1, BUFFER_SIZE, in);
if (EVP_EncryptUpdate(ctx, outbuf, &outlen, inbuf, inlen) != 1) {
fclose(in);
fclose(out);
EVP_CIPHER_CTX_free(ctx);
printf("无法加密数据\n");
return;
}
fwrite(outbuf, 1, outlen, out);
} while (inlen == BUFFER_SIZE);
// 结束加密操作
if (EVP_EncryptFinal_ex(ctx, outbuf, &outlen) != 1) {
fclose(in);
fclose(out);
EVP_CIPHER_CTX_free(ctx);
printf("无法结束加密操作\n");
return;
}
fwrite(outbuf, 1, outlen, out);
// 释放资源
fclose(in);
fclose(out);
EVP_CIPHER_CTX_free(ctx);
}
void decrypt_file_des(const char* input_file, const char* output_file, const unsigned char* key, const unsigned char* iv) {
// 打开输入文件
FILE* in = fopen(input_file, "rb");
if (in == NULL) {
printf("无法打开输入文件 %s\n", input_file);
return;
}
// 打开输出文件
FILE* out = fopen(output_file, "wb");
if (out == NULL) {
fclose(in);
printf("无法打开输出文件 %s\n", output_file);
return;
}
// 创建 DES 解密上下文
EVP_CIPHER_CTX* ctx = EVP_CIPHER_CTX_new();
if (ctx == NULL) {
fclose(in);
fclose(out);
printf("无法创建解密上下文\n");
return;
}
// 初始化 DES 解密上下文
if (EVP_DecryptInit_ex(ctx, EVP_des_cbc(), NULL, key, iv) != 1) {
fclose(in);
fclose(out);
EVP_CIPHER_CTX_free(ctx);
printf("无法初始化解密上下文\n");
return;
}
unsigned char inbuf[BUFFER_SIZE + EVP_MAX_BLOCK_LENGTH];
unsigned char outbuf[BUFFER_SIZE];
int inlen, outlen;
// 循环读取、解密和写入文件数据
do {
inlen = fread(inbuf, 1, BUFFER_SIZE + EVP_MAX_BLOCK_LENGTH, in);
if (EVP_DecryptUpdate(ctx, outbuf, &outlen, inbuf, inlen) != 1) {
fclose(in);
fclose(out);
EVP_CIPHER_CTX_free(ctx);
printf("无法解密数据\n");
return;
}
fwrite(outbuf, 1, outlen, out);
} while (inlen == BUFFER_SIZE + EVP_MAX_BLOCK_LENGTH);
// 结束解密操作
if (EVP_DecryptFinal_ex(ctx, outbuf, &outlen) != 1) {
fclose(in);
fclose(out);
EVP_CIPHER_CTX_free(ctx);
printf("无法结束解密操作\n");
return;
}
fwrite(outbuf, 1, outlen, out);
// 释放资源
fclose(in);
fclose(out);
EVP_CIPHER_CTX_free(ctx);
}
```
使用示例:
```c
int main() {
// 128 位 AES 密钥和 IV
unsigned char aes_key[] = { 0x00, 0x01, 0x02, 0x03, 0x04, 0x05, 0x06, 0x07, 0x08, 0x09, 0x0a, 0x0b, 0x0c, 0x0d, 0x0e, 0x0f };
unsigned char aes_iv[] = { 0x10, 0x11, 0x12, 0x13, 0x14, 0x15, 0x16, 0x17, 0x18, 0x19, 0x1a, 0x1b, 0x1c, 0x1d, 0x1e, 0x1f };
// 64 位 DES 密钥和 IV
unsigned char des_key[] = { 0x00, 0x01, 0x02, 0x03, 0x04, 0x05, 0x06, 0x07 };
unsigned char des_iv[] = { 0x08, 0x09, 0x0a, 0x0b, 0x0c, 0x0d, 0x0e, 0x0f };
// 加密 AES 文件
encrypt_file_aes("input.txt", "output_aes.bin", aes_key, aes_iv);
// 解密 AES 文件
decrypt_file_aes("output_aes.bin", "input_aes.txt", aes_key, aes_iv);
// 加密 DES 文件
encrypt_file_des("input.txt", "output_des.bin", des_key, des_iv);
// 解密 DES 文件
decrypt_file_des("output_des.bin", "input_des.txt", des_key, des_iv);
return 0;
}
```
相关推荐
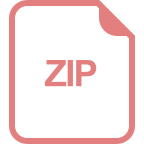
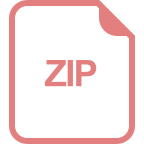














